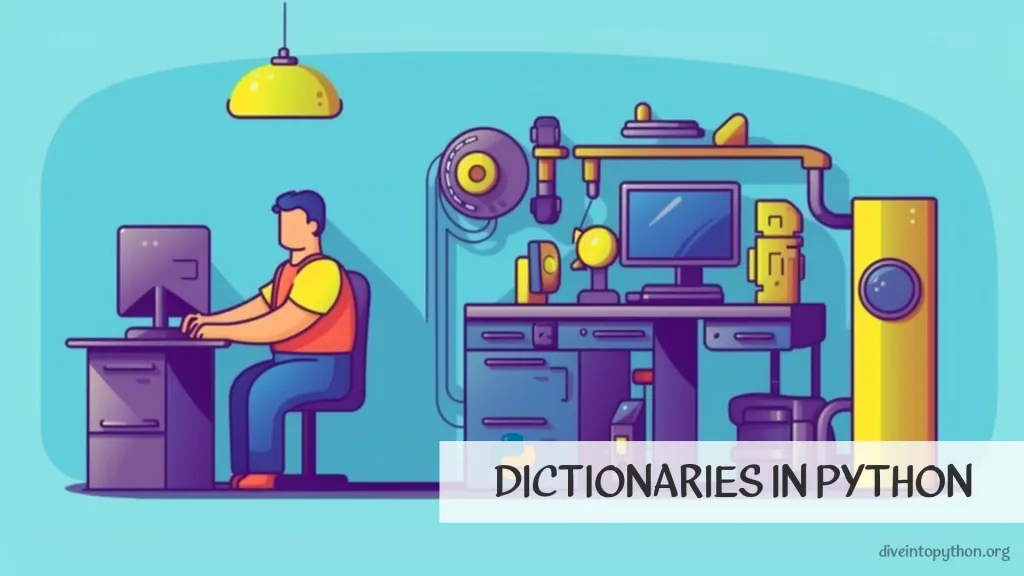
Python 提供了几种内置集合类型来存储和处理数据。Python 中一些常用的集合类型是
- 列表
- 元组
- 集合
- 字典
- 数组
- 双端队列
Python 中还有一些不太常用的集合类型,例如命名元组、默认字典和堆,它们是上面列出的基本集合类型的专门版本。
字典的定义
Python 中的字典是键值对的集合,其中每个键都与一个值关联。它是一个无序数据结构,字典中的每个键都必须是唯一的。
以下是 Python 中的字典示例
# Define a dictionary with key-value pairs
student = {
"name": "Alice",
"id": 12345,
"courses": ["Math", "Physics", "Chemistry"],
"grades": {"Math": 90, "Physics": 85, "Chemistry": 95}
}
如何在 Python 中创建字典
让我们看看如何在 Python 中创建或初始化字典。你可以使用大括号 {}
或使用 dict()
函数来创建字典。以下是一些示例
- 使用大括号
# Create an empty dictionary
empty_dict = {}
# Create a dictionary with key-value pairs
my_dict = {'name': 'John', 'age': 30, 'city': 'New York'}
# Create a dictionary with mixed data types
mixed_dict = {'name': 'John', 'age': 30, 'scores': [85, 90, 95]}
- 使用
dict()
函数
# Create an empty dictionary
empty_dict = dict()
# Create a dictionary with key-value pairs
my_dict = dict(name='John', age=30, city='New York')
# Create a dictionary with mixed data types
mixed_dict = dict(name='John', age=30, scores=[85, 90, 95])
在这两种情况下,字典都是使用一组键值对创建的。键必须是唯一且不可变的 数据类型(例如 字符串、数字 或 元组),而值可以是任何数据类型,包括 列表、字典和其他 对象。
删除字典
在 Python 中,你可以使用 del
关键字后跟字典变量名来删除字典。以下是一个示例
my_dict = {'key1': 'value1', 'key2': 'value2'}
del my_dict
在上面的示例中,我们创建了一个包含两个键值对的字典 my_dict
。del
关键字用于通过指定变量名 my_dict
来删除字典。
执行 del
语句 后,字典 my_dict
将从内存中完全删除,并且任何尝试访问它的操作都将导致 NameError
,因为该变量不再存在。
Python 中的字典访问
你可以使用其键从字典中获取值。以下是一个示例
# Creating a dictionary
my_dict = {'apple': 3, 'banana': 5, 'orange': 2}
# Accessing a value using its key
print(my_dict['apple']) # Output: 3
print(my_dict['banana']) # Output: 5
你还可以使用 get()
方法获取与字典中的键关联的值。以下是一个示例
# create a dictionary
my_dict = {'apple': 1, 'pineapple': 2, 'orange': 3}
# get the value associated with the 'apple' key
apple_value = my_dict.get('apple')
# print the value
print(apple_value) # Output: 1
如果在字典中找不到该键,get()
默认返回 None。
字典的字典
你可以通过简单地将字典用作另一个字典中的值来将字典嵌套到字典中。以下是一个示例
# create a dictionary of dictionaries
my_dict = {
'person1': {'name': 'John', 'age': 28},
'person2': {'name': 'Jane', 'age': 32}
}
# accessing values in the dictionary of dictionaries
print(my_dict['person1']['name']) # output: 'John'
print(my_dict['person2']['age']) # output: 32
要访问嵌套字典中的值,我们使用两组方括号。第一组方括号用于访问外部字典,第二组方括号用于访问内部字典。
你还可以像这样向嵌套字典中添加新字典
# adding a new person to the dictionary of dictionaries
my_dict['person3'] = {'name': 'Alex', 'age': 25}
# accessing the new person's information
print(my_dict['person3']['name']) # output: 'Alex'
同样,你可以在嵌套字典中更新值
# create a dictionary of dictionaries
my_dict = {
'person1': {'name': 'John', 'age': 28},
'person2': {'name': 'Jane', 'age': 32}
}
# updating a person's age in the dictionary of dictionaries
my_dict['person1']['age'] = 30
# accessing the updated age
print(my_dict['person1']['age']) # output: 30
总体而言,在 Python 中组合字典是存储和访问分层数据结构的一种简单而强大的方法。
Python 中对象的字典
这个主题与嵌套字典非常相似 - 你可以通过使用对象实例作为值并使用唯一键来访问对象来创建对象字典。以下是一个示例
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
# create objects
person1 = Person("Alice", 25)
person2 = Person("Bob", 30)
person3 = Person("Charlie", 35)
# create dictionary of objects
people = {
"person1": person1,
"person2": person2,
"person3": person3
}
# access objects using keys
print(people["person1"].name) # outputs "Alice"
print(people["person2"].age) # outputs 30
字典中键和值的逆转
让我们回顾一下如何在 Python 中逆转字典中的键和值。为此,你可以使用字典解析,并交换每个键值对中的键和值
my_dict = {'a': 1, 'b': 2, 'c': 3}
reversed_dict = {value: key for key, value in my_dict.items()}
print(reversed_dict) # Output: {1: 'a', 2: 'b', 3: 'c'}
在上面的代码中,我们首先定义了一个包含一些键值对的字典 my_dict
。然后,我们使用字典解析创建了一个新字典 reversed_dict
。在解析中,我们使用 items()
方法迭代 my_dict
字典,该方法返回一个 (键、值) 对列表。对于每个键值对,我们交换键和值的位置,并将新的 (值、键) 对添加到 reversed_dict
。
深入了解主题
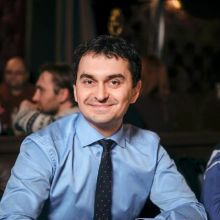

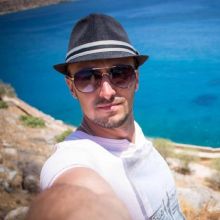