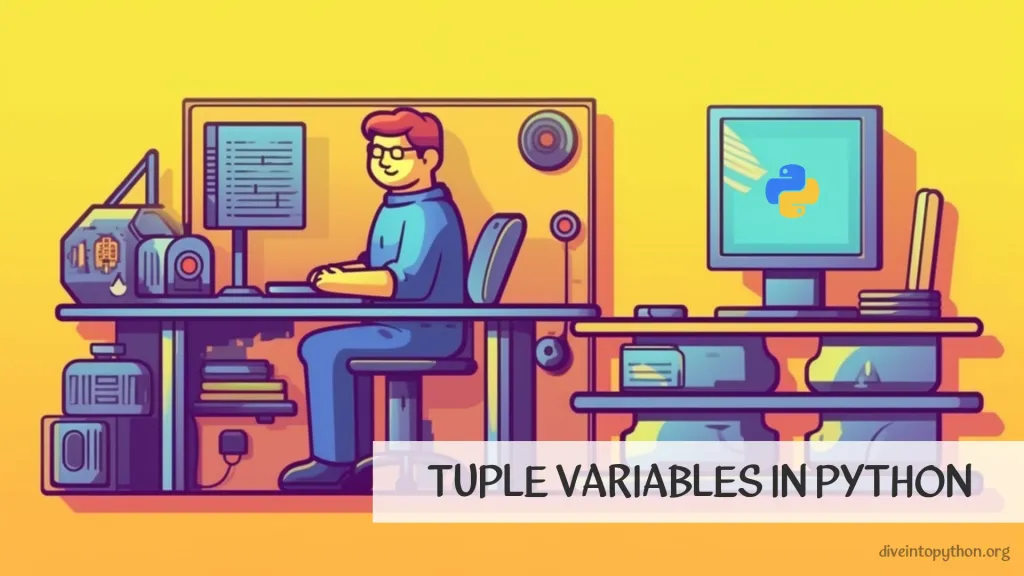
什么是 Python 中的元组?Python 中的元组是一个不可变的值序列,类似于列表。但是,元组一旦创建就不能修改,这意味着你不能在元组中添加、删除或更改元素。
在 Python 中创建元组
要创建元组,你可以使用括号 ()
并用逗号 ,
分隔元素。
以下是如何在 Python 中创建包含三个元素的元组的示例
my_tuple = (1, "hello", 3.14)
你还可以使用空括号 () 创建一个空元组
empty_tuple = ()
如果你想创建一个仅包含一个元素的元组,你需要在元素后添加一个逗号,因为如果没有逗号,Python 会将括号解释为分组运算符,而不是元组
single_tuple = (1,)
元组索引
你可以使用索引访问 Python 中的元组元素,就像在 列表 中一样。
正如我们已经提到的,Python 中的索引从 0 开始。这意味着元组中的第一个元素的索引为 0,第二个元素的索引为 1,依此类推。负索引也受支持,这意味着元组中的最后一个元素的索引为 -1,倒数第二个元素的索引为 -2,依此类推。
以下是如何使用索引访问元组元素的示例
my_tuple = ('apple', 'banana', 'cherry')
print(my_tuple[0]) # Output: 'apple'
print(my_tuple[1]) # Output: 'banana'
print(my_tuple[-1]) # Output: 'cherry'
如何在 Python 中追加到元组
在 Python 中,元组是不可变的,这意味着一旦创建,它们的元素就不能更改。但是,你可以通过组合现有元组和其他元素来创建一个新的元组。因此,要“追加”或“添加”一个元素到元组,你实际上会创建一个新的元组,其中包括现有元素和新元素。以下有几种实现此目的的方法
使用 +
运算符创建一个新的元组:
existing_tuple = (1, 2, 3)
new_element = 4
# Concatenate the existing tuple with a new tuple containing the new element
new_tuple = existing_tuple + (new_element,)
print(new_tuple)
使用 +=
增强赋值运算符:
existing_tuple = (1, 2, 3)
new_element = 4
# Use the += operator to concatenate the existing tuple with a new tuple containing the new element
existing_tuple += (new_element,)
print(existing_tuple)
这两种方法都通过将现有元组的元素与新元素组合来创建一个新的元组,有效地将元素“追加”到元组。请记住,元组是不可变的,所以你实际上是在创建一个新的元组,而不是修改原始元组。
元组的有序列表
你可以使用 sorted 函数对 Python 中的元组列表进行排序,并传递一个 key 参数,该参数指定如何比较每个元组中的元素。以下是一个示例
# define a list of tuples
my_list = [(1, 2), (3, 1), (2, 4)]
# sort the list by the first element in each tuple
sorted_list = sorted(my_list, key=lambda x: x[0])
print(sorted_list) # Output: [(1, 2), (2, 4), (3, 1)]
你还可以通过将 reverse 参数设置为 True 来按相反的顺序对元组列表进行排序
# define a list of tuples
my_list = [(1, 2), (3, 1), (2, 4)]
# sort the list by the second element in each tuple in reverse order
sorted_list = sorted(my_list, key=lambda x: x[1], reverse=True)
print(sorted_list) # Output: [(2, 4), (1, 2), (3, 1)]
Python 中的命名元组
命名元组是内置元组数据类型的子类,它允许通过名称和索引位置访问字段。
命名元组是使用 collections.namedtuple
函数创建的。以下是一个示例
from collections import namedtuple
# create a named tuple with two fields: 'x' and 'y'
Point = namedtuple('Point', ['x', 'y'])
# create an instance of the named tuple
p = Point(1, 2)
# access fields by index
print(p[0]) # prints 1
# access fields by name
print(p.x) # prints 1
print(p.y) # prints 2
命名元组类似于常规元组,但具有额外的优点,即具有命名字段,这可以使代码更具可读性和自文档性。它们与常规元组一样是不可变的,因此一旦创建,它们的字段就不能更改。
Python 中列表中的元组
在某些情况下,我们需要制作一个元组列表,例如对相关数据进行分组。因此,你可以使用以下语法创建一个包含元组的列表
my_list = [(item1, item2), (item3, item4), (item5, item6)]
列表中的每个元组可以包含多个项,这些项可以是任何数据类型,包括字符串、数字和其他元组。下面是包含具有不同数据类型的元组的列表示例
my_list = [("apple", 2), ("orange", 3), ("banana", 4.5), ("grape", ("red", "green"))]
你可以使用索引访问列表中元组中的项。例如,要访问上面列表中第一个元组中的第二个项,你可以使用以下代码
print(my_list[0][1]) # Output: 2
追加元组
要在 Python 中将元组追加到列表,可以使用 append()
方法。下面是一个示例
my_list = [(1, 2), (3, 4)]
new_tuple = (5, 6)
my_list.append(new_tuple)
print(my_list) # Output: [(1, 2), (3, 4), (5, 6)]
深入了解该主题
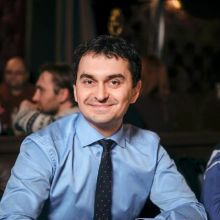

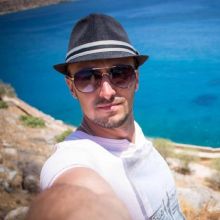