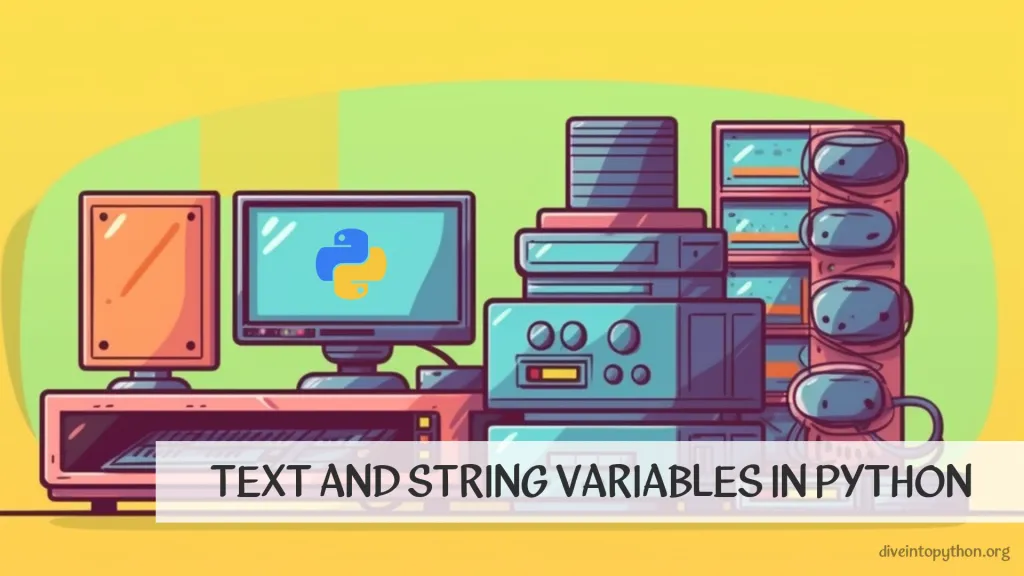
之前我们已经接触过 变量 的操作主题。在本部分中,我们将深入了解字符串最常见的操作和方法。这不是字符串操作的全部列表。
字符串变量声明
你可以通过使用等号 =
将字符串值分配给变量名来声明一个字符串变量。这是一个示例
my_string = "Hello, world!"
在此示例中,我们声明了一个名为 my_string
的字符串变量,并为其分配了值 "Hello, world!"
。请注意,字符串值用引号引起来。
你还可以通过将空字符串值分配给变量名来声明一个空字符串变量,如下所示
my_string = ""
在此示例中,我们声明了一个名为 my_string
的字符串变量,并为其分配了一个空字符串值。
字符串连接
字符串连接是将两个或多个字符串组合成一个字符串的过程。在 Python 中,你可以使用 +
运算符连接字符串。附加字符串的另一种选择是使用 +=
运算符。
我们来看一个附加字符串的示例
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print(result) # Output: Hello World
在上面的示例中,我们创建了两个字符串变量 str1
和 str2
,分别包含字符串 "Hello" 和 "World"。然后,我们使用 +
运算符将一个字符串添加到另一个字符串,同时添加一个空格字符,以创建一个单个字符串结果。最后,我们将添加结果打印到控制台,输出 "Hello World"。
字符串中的变量
在 Python 中,你可以使用不同的方法在字符串中包含变量。以下是一些常用的方法
字符串连接:你可以使用 +
运算符连接字符串和变量
name = "Alice"
age = 30
message = "Hello, my name is " + name + " and I am " + str(age) + " years old."
print(message)
使用 str.format()
方法:此方法允许你使用占位符 {}
在字符串中嵌入变量
name = "Bob"
age = 25
message = "Hello, my name is {} and I am {} years old.".format(name, age)
print(message)
使用 f 字符串(格式化字符串文字):在 Python 3.6 中引入,f 字符串提供了一种简洁的方法,可以通过在字符串前加上 f
并使用 {}
括起变量来直接在字符串中嵌入变量
name = "Charlie"
age = 35
message = f"Hello, my name is {name} and I am {age} years old."
print(message)
所有这些方法都可以实现相同的结果,但 f 字符串通常更受欢迎,因为它们具有可读性和易用性。它们允许你在字符串中直接引用变量,从而使代码更简洁、更易于理解。
字符串长度
你可以使用内置函数 len()
获取字符串的长度。你还可以使用此方法检查字符串是否为空。
以下是如何计算字符串包含的字符数的示例
my_string = "Hello, world!"
print(len(my_string)) # Output: 13
在此示例中,len()
函数返回 my_string
变量中字符的数量,即 13。你还可以使用 len()
来获取空字符串变量或包含空格的字符串变量的长度
empty_string = ""
print(len(empty_string)) # Output: 0
whitespace_string = " "
print(len(whitespace_string)) # Output: 3
在这两种情况下,len()
函数都会返回字符串的长度,对于空字符串为 0,对于包含空格的字符串为 3。
字符串比较
在 Python 中,你可以使用各种比较运算符来比较字符串。以下是一些示例
==
运算符:检查两个字符串是否相等。
str1 = "mango"
str2 = "pineapple"
if str1 == str2:
print("The two strings are equal.")
else:
print("The two strings are not equal.")
# Output: The two strings are not equal.
!=
运算符:检查两个字符串是否不相等。
str1 = "mango"
str2 = "pineapple"
if str1 != str2:
print("The two strings are not equal.")
else:
print("The two strings are equal.")
# Output: The two strings are not equal.
<
运算符:检查第一个字符串在词典顺序中是否小于第二个字符串。
str1 = "mango"
str2 = "pineapple"
if str1 < str2:
print("The first string comes before the second string.")
else:
print("The second string comes before the first string.")
# Output: The first string comes before the second string.
>
运算符:检查第一个字符串在词典顺序中是否大于第二个字符串。
str1 = "mango"
str2 = "pineapple"
if str1 > str2:
print("The first string comes after the second string.")
else:
print("The second string comes after the first string.")
# Output: The second string comes after the first string.
<=
运算符:检查第一个字符串是否小于或等于第二个字符串(按字典顺序)。
str1 = "mango"
str2 = "pineapple"
if str1 <= str2:
print("The first string comes before or is equal to the second string.")
else:
print("The second string comes before the first string.")
# Output: The first string comes before or is equal to the second string.
>=
运算符:检查第一个字符串是否大于或等于第二个字符串(按字典顺序)。
str1 = "mango"
str2 = "pineapple"
if str1 >= str2:
print("The first string comes after or is equal to the second string.")
else:
print("The second string comes after the first string.")
# Output: The second string comes after the first string.
请注意,在 Python 中比较字符串时,比较是按字典顺序进行的,这意味着比较基于字符串中字符的 ASCII 值。
多行字符串
您可以通过将文本括在三引号中来创建多行字符串,即三个单引号 (''') 或三个双引号 (""").
例如
multiline_string = '''
This is a multiline string
that spans multiple lines.
You can use single quotes
or double quotes.
'''
print(multiline_string)
# Output:
This is a multiline string
that spans multiple lines.
You can use single quotes
or double quotes.
请注意,三引号字符串中的任何空白,包括换行符,都将包含在字符串中。如果您想排除行首或行尾的空白,可以使用 strip() 或 rstrip() 等字符串方法。
二进制字符串
可以使用前缀 0b
后跟一系列 0
和 1
数字来表示二进制字符串。例如,二进制字符串 1101
可以表示为 0b1101
。以下是一些在 Python 中创建和操作二进制字符串的示例
- 创建二进制字符串
binary_str = '0b1101'
- 将十进制整数转换为二进制字符串
decimal_num = 13
binary_str = bin(decimal_num)
- 将二进制字符串转换为十进制整数
binary_str = '0b1101'
decimal_num = int(binary_str, 2)
- 二进制字符串上的按位运算
binary_str1 = '0b1101'
binary_str2 = '0b1010'
# Bitwise AND
result = int(binary_str1, 2) & int(binary_str2, 2)
print(bin(result)) # Output: 0b1000
# Bitwise OR
result = int(binary_str1, 2) | int(binary_str2, 2)
print(bin(result)) # Output: 0b1111
# Bitwise XOR
result = int(binary_str1, 2) ^ int(binary_str2, 2)
print(bin(result)) # Output: 0b0111
请注意,在执行按位运算时,我们需要使用 int()
函数将二进制字符串转换为十进制 整数,其中第二个参数指定基数(在本例中为 2
),然后使用 bin()
函数将结果转换回二进制字符串。
在字符上迭代
有几种方法可以在 Python 中遍历字符串中的字符。以下是一些示例
- 使用
for
循环
my_string = "Hello, world!"
for char in my_string:
print(char)
- 使用
while
循环
my_string = "Hello, world!"
i = 0
while i < len(my_string):
print(my_string[i])
i += 1
- 使用列表解析
my_string = "Hello, world!"
char_list = [char for char in my_string]
print(char_list)
- 使用
map()
函数
my_string = "Hello, world!"
char_list = list(map(str, my_string))
print(char_list)
- 使用
enumerate()
函数
my_string = "Hello, world!"
for index, char in enumerate(my_string):
print(f"Character at index {index}: {char}")
所有这些方法都允许您遍历字符串中的字符并对它们执行操作。选择最适合您需求的方法!
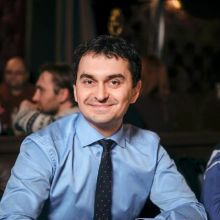

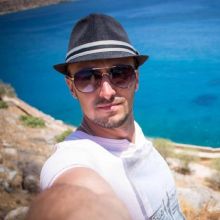