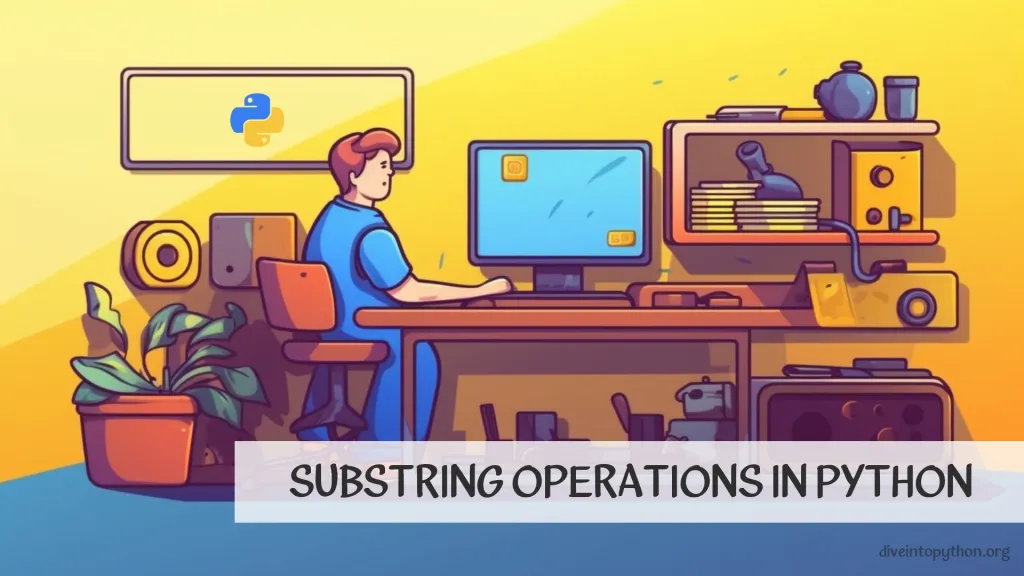
子字符串是较大字符串的一部分的字符序列。它是字符串中连续的字符序列,可以独立提取或操作。
例如,在字符串“Hello, World!”中,子字符串“Hello”、“World”和“!”都是原始字符串的子字符串。
让我们回顾一下与子字符串相关的最常见操作和方法。
字符串切片
字符串切片是从现有 Python 中的字符串 创建新子字符串的过程。你可以使用语法 [start:end]
切片字符串,以提取字符串中从 start
索引开始到 end
索引结束(不包括)的部分。这里有一些示例
my_string = "Hello, world!"
# Get the substring from index 0 to index 4 (not inclusive)
print(my_string[0:4]) # Output: "Hell"
# Get the substring from index 7 to the end of the string
print(my_string[7:]) # Output: "world!"
# Get the substring from index 2 to index 8 (not inclusive) with a step of 2
print(my_string[2:8:2]) # Output: "lo,"
# Get the substring from index 2 to the second-to-last character
print(my_string[2:-1]) # Output: "llo, world"
在第一个示例中,我们使用切片从 my_string
变量中提取子字符串 “Hell”
,方法是指定起始索引 0
和结束索引 4
(不包括)。
在第二个示例中,我们使用切片从 my_string
变量中提取子字符串 “world!”
,方法是仅指定起始索引 7
并将结束索引留空。这告诉 Python 从起始索引提取子字符串到字符串的末尾。
在第三个示例中,我们使用切片从子字符串 “lo, ”
中提取每个字符。我们通过指定起始索引 2
、结束索引 8
(不包括)和步长 2
来执行此操作。
在第四个示例中,我们使用切片从 my_string
变量中提取子字符串 “llo, world”
,方法是指定起始索引 2
和结束索引 -1
。-1
指定字符串中倒数第二个字符作为结束索引。
要使用分隔符拆分字符串,你还可以使用 split()
方法。此方法接受一个参数,即你希望用来拆分字符串的分隔符字符或字符串。这是一个示例
my_string = "Hello,world"
my_list = my_string.split(",")
print(my_list) # Output: ['Hello', 'world']
你可以使用字符串切片从 Python 中的字符串中删除第一个字符。这是一个示例
string = "hello"
new_string = string[1:]
print(new_string) # Output: ello
字符串切片还可以用来从字符串中删除最后一个字符。这是一个示例
my_string = "Hello World!"
new_string = my_string[:-1]
print(new_string) # Output: "Hello World"
字符串子集
要检查 Python 字符串是否包含特定子字符串,你可以使用 in
关键字或 find()
方法。
下面是一个使用 in 关键字的示例
my_string = "Hello, world!"
if "world" in my_string:
print("Substring found!")
else:
print("Substring not found.")
# Output: Substring found!
下面是一个使用 find()
方法的示例
my_string = "Hello, world!"
if my_string.find("world") != -1:
print("Substring found!")
else:
print("Substring not found.")
# Output: Substring found!
在这两个示例中,我们检查子字符串 “world”
是否存在于字符串 my_string
中。如果找到子字符串,我们打印 “Substring found!”
,否则我们打印 “Substring not found.”
。
字符串反转
有几种方法可以在 Python 中反转字符串。
让我们看看如何使用示例在 python 中反转字符串:
- 使用切片
string = "hello"
reversed_string = string[::-1]
print(reversed_string) # Output: "olleh"
- 使用循环
string = "hello"
reversed_string = ""
for char in string:
reversed_string = char + reversed_string
print(reversed_string) # Output: "olleh"
- 使用 reversed() 函数
string = "hello"
reversed_string = "".join(reversed(string))
print(reversed_string) # Output: "olleh"
所有这些方法都将产生相同的结果,即原始字符串的反转版本。
startswith() 和 endswith() 方法
在 Python 中,startswith()
和 endswith()
是两个字符串方法,分别用于检查字符串是否以特定前缀或后缀开头或结尾。以下是这些方法的概述
startswith()
方法用于检查字符串是否以特定前缀开头。该方法将一个或多个前缀作为参数,如果字符串以其中任何一个前缀开头,则返回 True
,否则返回 False
。以下是 startswith()
方法的语法
string.startswith(prefix, start=0, end=len(string))
其中
-
prefix
是要检查的前缀。 -
start
是一个可选参数,指定要搜索的字符串的起始索引。默认情况下,start
设置为 0,这意味着将搜索整个字符串。 -
end
是一个可选参数,指定要搜索的字符串的结束索引。默认情况下,end
设置为字符串的长度。
下面是使用 startswith() 方法的一个示例
s = "Python is a great programming language"
print(s.startswith("Python")) # True
print(s.startswith("Java")) # False
print(s.startswith(("Java", "Python"))) # True (checking multiple prefixes)
endswith()
方法用于检查字符串是否以特定后缀结尾。该方法将一个或多个后缀作为参数,如果字符串以其中任何一个结尾,则返回 True
,否则返回 False
。以下是 endswith()
方法的语法
string.endswith(suffix, start=0, end=len(string))
其中
-
suffix
是要检查的后缀。 -
start
和end
参数与startswith()
方法中的含义相同。
下面是使用 endswith()
方法的一个示例
s = "Python is a great programming language"
print(s.endswith("language")) # True
print(s.endswith("Python")) # False
print(s.endswith(("Python", "language"))) # True (checking multiple suffixes)
在这两种方法中,你可以传递一个前缀或后缀元组来检查多种可能性。start
和 end
参数是可选的,可用于仅搜索字符串的一部分。
split() 方法
它也是 Python 中的一个内置方法,用于根据指定的分隔符将字符串拆分和解析为子字符串列表。默认情况下,使用的分隔符是空格。
下面是一个示例
s = "Hello World! How are you?"
words = s.split()
print(words) # Output: ['Hello', 'World!', 'How', 'are', 'you?']
在上面的示例中,split()
方法在字符串 s
上调用,其中包含空格分隔的单词。结果列表 words 包含原始字符串中的所有单词作为单独的元素。
你还可以使用 split()
方法指定不同的分隔符。例如
s = "mango,pineapple,banana"
fruits = s.split(",")
print(fruits) # Output: ['mango', 'pineapple', 'banana']
在此示例中,split()
方法在字符串 s
上调用,其中包含逗号分隔的水果名称。结果列表 fruits 包含所有水果名称作为单独的元素,其中逗号用作分隔符。
string.find() 函数
这是内置 Python 函数,它返回给定字符串中子字符串首次出现的索引。如果找不到子字符串,它将返回 -1
。使用 string.find()
的语法如下
string.find(substring, start=0, end=len(string))
其中 string
是要搜索的字符串,substring
是要搜索的字符串,start
是搜索的起始索引(默认为 0),end
是搜索的结束索引(默认为字符串的长度)。
这里有一个示例
sentence = "The quick brown fox jumps over the lazy dog"
print(sentence.find("fox")) # Output: 16
print(sentence.find("cat")) # Output: -1
在第一行中,我们定义了一个字符串 sentence。然后,我们使用 find()
函数在 sentence
字符串中搜索子字符串 "fox"
。由于 "fox"
在 sentence
字符串中的索引 16 处找到,因此 find()
函数返回 16
。在第二行中,我们搜索子字符串 "cat"
,它在 sentence
字符串中未找到,因此 find()
函数返回 -1
。
replace() 方法
在 Python 中,字符串是不可变的,这意味着一旦创建字符串,你就不能更改它。但是,你可以创建一个包含原始字符串修改版本的新字符串。
若要从 Python 中的字符串中删除特定字符或子字符串,可以使用 replace()
方法或字符串切片。
以下是如何使用 replace() 方法移除特定字符的示例
my_string = "Hello, World!"
new_string = my_string.replace("o", "")
print(new_string) # Output: Hell, Wrld!
在此,我们将字符 "o"
替换为空字符串,有效地从原始字符串中将其移除。
字符串截断
你可以通过指定想要保留的字符串的最大长度来截断字符串。
下面是一个示例
text = "This is a long text that needs to be truncated."
max_length = 20
truncated_text = text[:max_length] + "..." if len(text) > max_length else text
print(truncated_text) # Output: This is a long text...
在此示例中,我们首先定义一个想要截断的字符串 text
。我们还使用 max_length
变量指定截断字符串的最大长度。
然后,我们使用切片获取字符串的前 max_length
个字符。如果原始字符串的长度大于 max_length
,我们使用字符串连接在截断字符串的末尾追加省略号。如果原始字符串的长度小于或等于 max_length
,我们只需将原始字符串分配给 truncated_text
变量。
最后,我们使用 print()
函数打印截断字符串。
count() 方法
你可以使用内置的 count
方法计算字符串中子字符串出现的次数。
以下是子字符串 "is"
的计数器
string = "Hello, world! This is a sample string."
substring = "is"
count = string.count(substring)
print(count) # Output: 2
在此示例中,我们有一个字符串 "Hello, world! This is a sample string."
,我们想要计算子字符串 "is"
出现的次数。我们使用 count 方法计算子字符串在字符串中出现的次数。
此程序的输出将为 2
,因为子字符串 "is"
在字符串中出现两次。
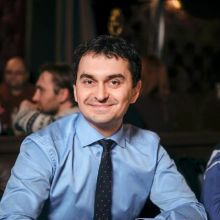

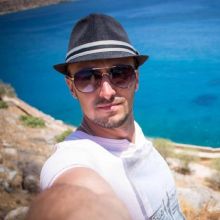