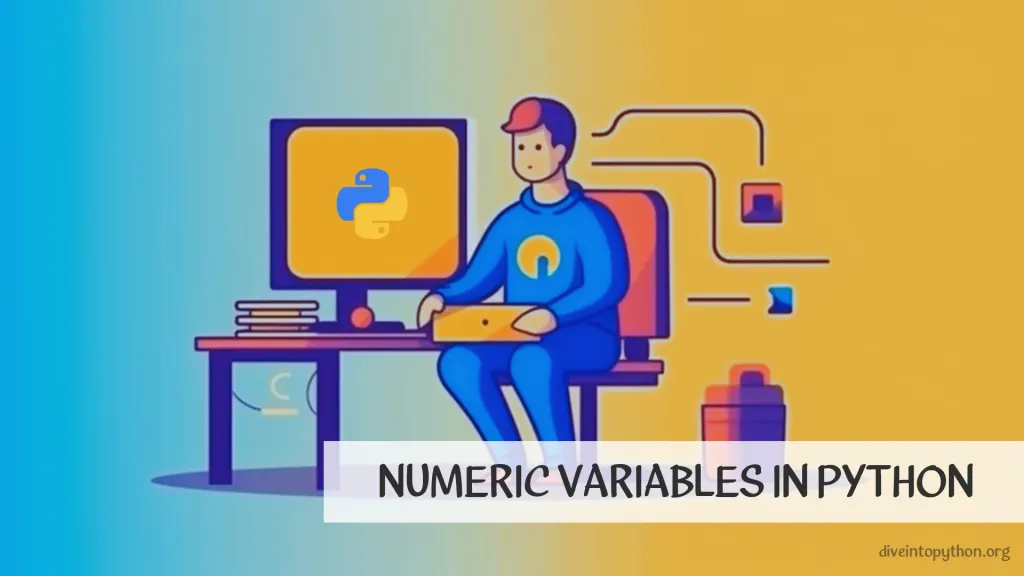
Python 支持多种数字 数据类型,这些数据类型用于编程中的各种数学运算。这些数据类型包括整数、浮点数和复数。对于任何想要在 Python 中处理数字数据的程序员来说,了解这些数据类型及其特征至关重要。
Python 中的数字类型
在 Python 中,有几种数字类型可用于不同的目的。Python 中最常见的数字类型是
-
整数 (int):这是一个没有小数点的整数。例如,
1
、2
、3
等是整数。 -
浮点数:这是一个小数。例如,
1.2
、3.14159
等是浮点数。 -
复数:这是一个具有实部和虚部的数字。例如,
1 + 2j
、3.14 - 4j
等是复数。
以下是如何在 Python 中定义这些数字类型的一些示例
# Integer
x = 5
print(type(x)) # Output: <class 'int'>
# Float
y = 3.14
print(type(y)) # Output: <class 'float'>
# Complex
z = 2 + 3j
print(type(z)) # Output: <class 'complex'>
isinstance() 函数
要检查变量在 Python 中是否为数字,可以使用 isinstance()
函数来检查变量是否属于 int、float 或 complex 数据类型。这是一个示例
x = 10
y = 3.14
z = 2 + 3j
print(isinstance(x, (int, float, complex))) # Output: True
print(isinstance(y, (int, float, complex))) # Output: True
print(isinstance(z, (int, float, complex))) # Output: True
print(isinstance('hello', (int, float, complex))) # Output: False
在 Python 中四舍五入数字
可以使用内置的 round()
函数对数字进行四舍五入。round()
函数有两个参数:要四舍五入的数字和小数位数。
这是一个示例
x = 3.14159
rounded_x = round(x, 2)
print(rounded_x) # Output: 3.14
请注意,如果要四舍五入的数字以 5 结尾,round()
函数将四舍五入到最接近的偶数。这称为“银行家四舍五入”。例如
x = 2.5
rounded_x = round(x)
print(rounded_x) # Output: 2
数字格式化
在 Python 中,有几种方法可以格式化数字。以下是一些示例
使用内置的 format()
函数
x = 3.14159
print("{:.2f}".format(x)) # Output: 3.14
format()
函数中的 "{:.2f}"
字符串告诉 Python 将数字格式化为小数点后两位的浮点数。
使用 f 字符串(Python 3.6 及更高版本)
x = 3.14159
print(f"{x:.2f}") # Output: 3.14
字符串前面的 f
表示这是一个 f 字符串,字符串中的 "{x:.2f}"
告诉 Python 将 x
的值格式化为小数点后两位的浮点数。
使用 %
运算符
x = 3.14159
print("%.2f" % x) # Output: 3.14
%
运算符中的 %.2f
字符串告诉 Python 将数字格式化为小数点后两位的浮点数。
检查字符串是否为数字
可以使用多种方法检查字符串在 Python 中是否为数字。以下是一些示例
使用 isnumeric()
方法
my_string = "123"
if my_string.isnumeric():
print("String is a number")
else:
print("String is not a number") # Output: String is a number
使用 isdigit()
方法
my_string = "456"
if my_string.isdigit():
print("String is a number")
else:
print("String is not a number") # Output: String is a number
使用 try-except 块将字符串转换为浮点数
my_string = "789.12"
try:
float(my_string)
print("String is a number")
except ValueError:
print("String is not a number") # Output: String is a number
请注意,如果字符串包含非数字字符,前两种方法将返回
False
,第三种方法将引发ValueError
。
检查数字是否为整数
你可以使用模运算符 %
来检查一个数字是否为整数。如果数字除以 1 的结果等于 0,则该数字为整数。
这是一个示例
num = 5.0 # the number you want to check
if num % 1 == 0:
print("The number is whole.")
else:
print("The number is not whole.") # Output: "The number is whole."
生成随机数
要在 Python 中获取一个随机数,你可以使用 random 模块。以下是如何生成一个 0 到 10 之间的随机整数的示例
import random
random_number = random.randint(0, 10)
print(random_number)
每次运行脚本时,这都将打印一个 0 到 10(包括 0 和 10)之间的随机整数。如果你想生成一个随机浮点数,你可以使用 random.uniform()
函数
import random
random_number = random.uniform(0, 1)
print(random_number)
在 Python 中对数字求平方
我们来看看如何在 Python 中对一个数字求平方。第一种方法是使用指数运算符 **
。以下是一个示例
x = 5
squared = x ** 2
print(squared) # Output: 25
或者,你也可以使用 pow()
函数来计算一个数字的平方。以下是一个示例
x = 5
squared = pow(x, 2)
print(squared)
对数字取反
你可以使用 -
(减号)运算符对一个数字取反。
这是一个示例
number = 10
negated_number = -number
print(negated_number) # Output: -10
素数
素数是大于 1 的正整数,除了 1 和它本身之外,没有其他正整数因子。在 Python 中,你可以通过测试一个数字是否可以被 1 和它本身之外的任何数字整除来检查它是否为素数。
以下是一个检查一个数字是否为素数的函数示例
def is_prime(n):
if n <= 1:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
此函数接受一个正整数 n
作为输入,如果 n
为素数,则返回 True
,否则返回 False
。
该函数首先检查 n
是否小于或等于 1,根据定义,这不是素数。如果 n
小于或等于 1,则该函数返回 False
。
如果 n
大于 1,则该函数检查它是否可以被 2 到 n
的平方根之间的任何数字整除。如果 n
可以被此范围内的任何数字整除,则它不是素数,并且该函数返回 False
。否则,n
是素数,并且该函数返回 True
。
以下是如何使用 is_prime
函数的示例
print(is_prime(7)) # True
print(is_prime(15)) # False
print(is_prime(23)) # True
print(is_prime(1)) # False
输出
True
False
True
False
在此示例中,我们使用不同的输入值调用了 is_prime
函数并打印了输出。
Python 中的欧拉数
欧拉数,也称为数学常数 e
,是一个数学常数,大约等于 2.71828。在 Python 中,你可以使用 math
模块计算欧拉数。
以下是如何计算欧拉数的示例
import math
e = math.e
print(e) # Output: 2.718281828459045
从字符串中提取数字
要从 Python 中的字符串 中提取一个数字,你可以使用带 re
模块的正则表达式。以下是一个示例
import re
string = "The price is $12.34"
number = re.findall(r'\d+\.\d+', string)[0]
print(number) # Output: 12.34
在此示例中,我们首先导入了 re
模块,它提供了对正则表达式的支持。然后我们定义了一个 string
变量 string,其中包含一个带有数字的句子。
要从字符串中提取数字,我们使用正则表达式模式 \d+\.\d+
使用 re.findall
函数。此模式匹配一个或多个数字 \d+
,后跟一个点 \.
,后跟一个或多个数字 \d+
。结果匹配是一个字符串,表示句子中的数字。
由于 re.findall
返回一个匹配列表,我们访问了列表的第一个元素 [0]
以获取数字作为字符串。
如果您需要将从字符串中提取的数字转换为数值,可以使用 float 或 int 函数
number = float(number)
print(number) # Output: 12.34
数字的位数
要在 Python 中查找数字的位数,可以将数字转换为字符串,然后遍历字符串以提取每个位数。以下是一个示例
num = 12345
# Convert number to string
num_str = str(num)
# Iterate over string and print each digit
for digit in num_str:
print(digit)
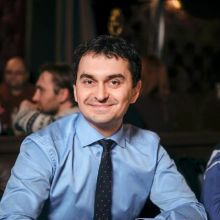

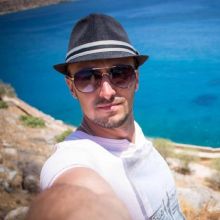