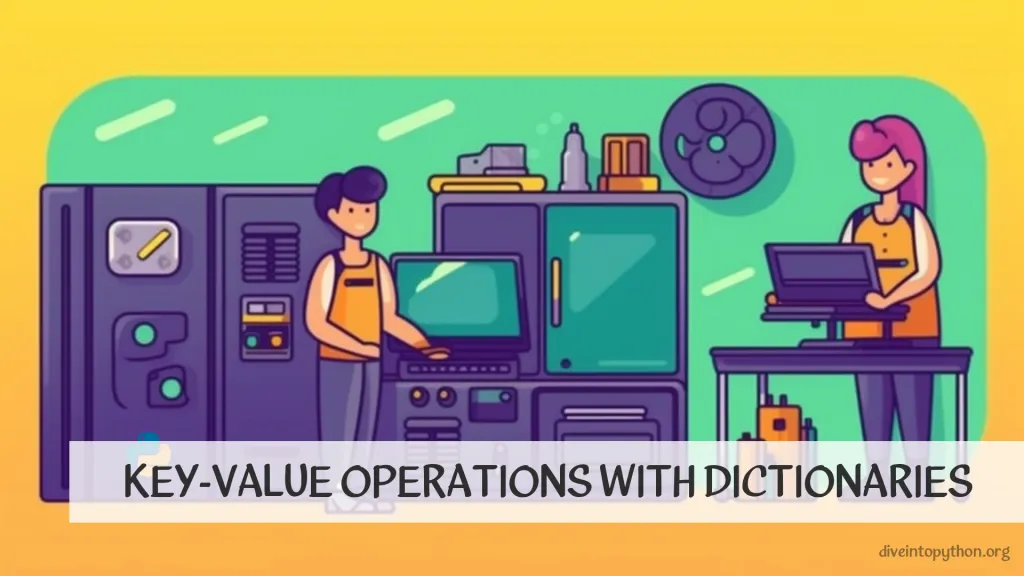
我们经常会遇到需要使用字典的键或值的情况。幸运的是,字典提供了各种操作来处理键和值。
获取字典键
要获取 Python 中的字典键,可以使用 keys()
方法。以下是获取键集的一个示例
# Creating a dictionary
my_dict = {"name": "Sam", "age": 35, "city": "Boston"}
# Getting the keys of the dictionary
keys = my_dict.keys()
# Printing the keys
print(keys) # Output: dict_keys(['name', 'age', 'city'])
请注意,keys()
返回一个视图对象,该对象是字典键的动态视图。这意味着如果修改了字典,视图对象将反映这些更改。如果你需要将键作为列表来处理,可以将视图对象转换为列表。
将字典键转换为列表
要将 Python 中的字典键转换为列表,可以使用 keys()
方法和 list()
函数。以下是一个示例
my_dict = {'a': 1, 'b': 2, 'c': 3}
keys_list = list(my_dict.keys())
print(keys_list) # Output: ['a', 'b', 'c']
在上面的代码中,my_dict.keys()
返回一个包含 my_dict
键的 dict_keys
对象。list()
函数用于将此对象转换为列表,该列表被分配给变量 keys_list
。最后,keys_list
被打印到控制台。
检查键是否存在
在 Python 中,字典不可用 has_key()
方法。相反,你可以使用 in
运算符来检查字典是否具有键。让我们看看如何在 Python 中的字典 中检查键是否存在
# Creating a dictionary
my_dict = {"name": "Sam", "age": 35, "city": "Boston"}
# Checking if a key is present in the dictionary
if "name" in my_dict:
print("The key 'name' is present in the dictionary")
else:
print("The key 'name' is not present in the dictionary")
if "country" in my_dict:
print("The key 'country' is present in the dictionary")
else:
print("The key 'country' is not present in the dictionary")
这将输出
The key 'name' is present in the dictionary
The key 'country' is not present in the dictionary
请注意,in
运算符检查键是否存在于字典中,而不是键的值是否为 None
。如果你需要检查字典中键的值是否存在,可以使用 get()
方法。
从字典中删除键
在 Python 中,你可以使用 del 语句 或 pop() 方法从字典中删除键。
以下是一个使用 del 语句从字典中删除键的示例
my_dict = {"a": 1, "b": 2, "c": 3}
del my_dict["b"]
print(my_dict) # Output: {"a": 1, "c": 3}
在上面的示例中,键 "b"
使用 del
语句从 my_dict
字典中删除。
以下是一个使用 pop()
方法从字典中删除键的示例
my_dict = {"a": 1, "b": 2, "c": 3}
my_dict.pop("b")
print(my_dict) # Output: {"a": 1, "c": 3}
在上面的示例中,键 "b"
使用 pop()
方法从 my_dict
字典中删除。pop()
方法还返回与已删除键关联的值,如果你需要根据该值执行一些其他处理,这将很有用。
将字典值转换为列表
你可以使用 Python 字典的 values()
方法获取其值的列表。
以下是一个示例
my_dict = {'a': 1, 'b': 2, 'c': 3}
values_list = list(my_dict.values())
print(values_list) # Output: [1, 2, 3]
在上面的代码中,我们创建了一个具有三个键值对的字典 my_dict
。然后我们调用字典上的 values()
方法以获取其值的视图对象,并使用 list()
构造函数将其转换为列表。最后,我们打印结果值列表。
请注意,列表中值的顺序不能保证与字典中键的顺序相同,因为字典在 Python 中是无序的。
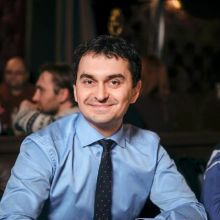

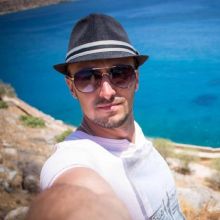