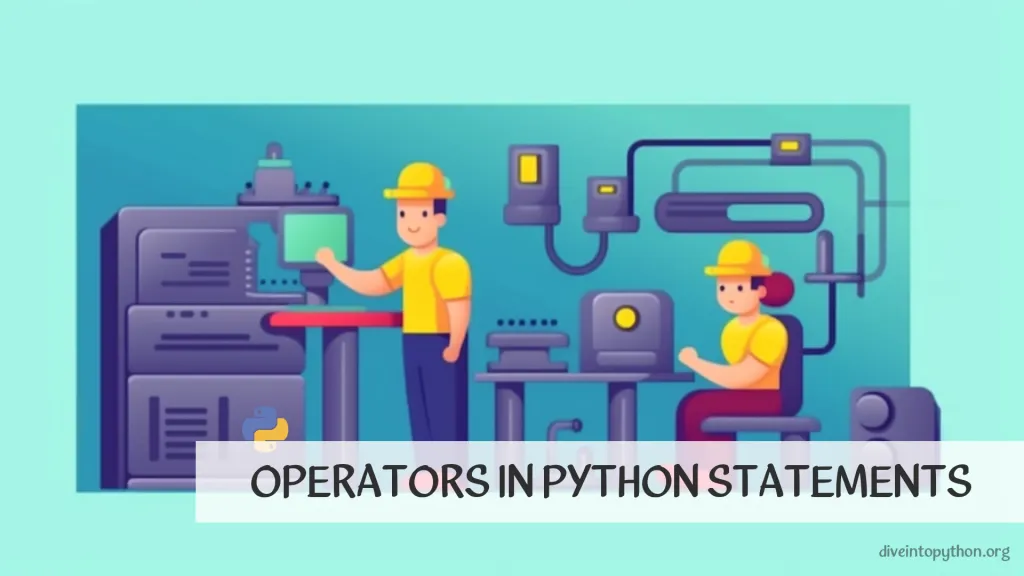
在本文中,我们将探讨 Python 中不同类型的运算符以及它们在编程中的用法。
in 运算符
Python 中的 in
运算符用于检查序列中是否存在某个值。如果在序列中找到了该值,则返回布尔值 True
,否则返回 False
。
my_list = [1, 2, 3, 4, 5]
x = 3
if x in my_list:
print("Element is present in the list")
else:
print("Element is not present in the list")
# Output:
#
# Element is present in the list
my_string = "Hello World"
x = "o"
if x in my_string:
print("Substring is present in the string")
else:
print("Substring is not present in the string")
# Output:
#
# Substring is present in the string
使用 in
运算符,我们可以轻松检查 Python 中列表、元组或任何其他序列数据类型中元素的存在。它还有助于简化代码并使其更具可读性。
如何在 if 中使用 and 运算符
Python 中的 and
运算符用于 if
语句 中,以测试多个条件是否为真。如果两个条件都为真,则返回 True
,否则返回 False
。以下有两个示例
x = 5
y = 10
if x > 0 and y > 0:
print("Both x and y are positive")
else:
print("At least one of x and y is not positive")
在此示例中,and
运算符用于确定 x
和 y
是否都为正。由于 x
和 y
都大于 0,因此 if
语句求值为 True
,并打印 Both x and y are positive
。
name = "John"
age = 25
if name == "John" and age == 25:
print("Your name is John and you are 25 years old")
else:
print("You are not John or you are not 25 years old")
在此示例中,and
运算符用于检查 name
是否为 John
且 age
是否为 25。由于两个条件都为真,因此 if
语句求值为 True
,并打印 Your name is John and you are 25 years old
。
总之,Python 中的 and
运算符是 if
语句中一个强大的工具,它允许你一次检查多个条件。它在需要进行大量条件检查的复杂程序中非常有用。
or 运算符
Python 中的 or
运算符是一个逻辑运算符,如果两个操作数中的任何一个为 True
,则返回 True
,如果两个操作数都为 False
,则返回 False
。它可以在条件语句或布尔表达式中使用。
条件语句中的 or
运算符
age = 25
if age < 18 or age > 60:
print("You are not eligible for this job")
else:
print("You are eligible for this job")
# Output: `You are eligible for this job`
在此示例中,or
运算符用于检查 age
变量是否小于 18 或大于 60。如果任一条件为 True
,它将打印一条消息,说明该人不符合工作资格。否则,它将打印一条消息,说明该人符合工作资格。
布尔表达式中的 or
运算符
x = 5
y = 10
result = x > 3 or y < 9
print(result)
# Output: `True`
在此示例中,or
运算符用于布尔表达式中,以检查 x
是否大于 3 或 y
是否小于 9。由于 x
大于 3,因此表达式求值为 True
,并且 result
打印为 True
。
总体而言,Python 中的 or
运算符提供了一种简单的方法来检查布尔表达式中至少一个条件是否为 True
。
not 运算符
Python 中的 not
运算符用于反转布尔表达式的逻辑状态。如果表达式为 False
,则返回 True
;如果表达式为 True
,则返回 False
。这里有两个示例
如何将 not
与布尔变量一起使用
flag = False
print(not flag)
not
与比较运算符一起使用
x = 10
y = 5
print(not x > y) ### Output
总体而言,not
运算符是 Python 中一个有用的工具,用于更改布尔表达式的真值。它可以与布尔 变量 一起使用,或与比较运算符一起使用。
不等于运算符
Python 中的不等号运算符用于比较两个值,如果它们不相等,则返回 True
;如果它们相等,则返回 False
。不等号运算符使用的符号是 !=
。
value1 != value2
a = 5
b = 3
if a != b:
print("a is not equal to b")
# Output:
#
# a is not equal to b
name1 = "John"
name2 = "Mary"
if name1 != name2:
print("Names are not equal")
# Output:
#
# Names are not equal
通过在 Python 中使用不等号运算符,你可以轻松比较两个值并获得所需的输出。此运算符在需要检查两个值是否不相等的情况下非常有用。
除法
在 Python 中,有三种除法运算符:单正斜杠 /
运算符、双正斜杠 //
运算符和百分号 %
运算符。
/
运算符执行常规除法并返回浮点数答案,而 //
运算符执行向下取整除法并返回商的整数。%
运算符返回除法的余数。%
的另一个名称是模运算符
x = 10
y = 3
result = x / y
print(result)
输出:3.3333333333333335
x = 10
y = 3
floor_division = x // y
# Use modulo operator
remainder = x % y
print(floor_division)
print(remainder)
# Output:
#
# 3
# 1
总体而言,在处理数学运算时,了解这些运算符及其差异在 Python 编程中非常重要。
: 运算符
Python 中的 :
运算符用于切片序列,例如 列表、元组 和 字符串。它允许你通过指定用冒号分隔的起始索引和结束索引来提取序列的一部分。你还可以指定步长。此运算符在使用 Python 中的数据时非常有用。
### create a list
my_list = [0, 1, 2, 3, 4, 5]
### slice the list from index 2 to index 4
sliced_list = my_list[2:5]
### print the sliced list
print(sliced_list)
# Output:
#
# [2, 3, 4]
### create a string
my_string = "Hello world!"
### slice the string to get characters from index 1 to index 4
sliced_string = my_string[1:5]
### print the sliced string
print(sliced_string)
# Output:
#
# ello
:
运算符是 Python 中用于处理序列的基本工具。其灵活的语法使切片和切块数据变得轻而易举!
Python 中的布尔运算符
Python 中的布尔运算符用于评估真或假条件。这些运算符包括 and
、or
和 not
。在 Python 中,布尔运算使用关键字 and
、or
和 not
创建。
x = 5
y = 10
z = 15
if x < y and z > y:
print("Both conditions are true")
# Output: `Both conditions are true`
x = 5
y = 10
z = 15
if x < y or z < y:
print("At least one condition is true")
# Output: `At least one condition is true`
在 Python 中使用布尔运算符提供了评估多个条件和做出决策的灵活性。通过使用布尔运算,可以简化复杂的语句并用更少的代码行进行编码。
运算符重载
在 Python 中,运算符重载使我们能够为特定类或对象以自定义方式定义运算符的行为。这有助于提高代码的清晰度和可读性。Python 支持对大多数内置运算符进行运算符重载,例如 +
、-
、/
、//
、%
、&
、|
、^
、>>
、<<
、<
、>
、<=
、>=
、==
、!=
等。
以下是两个演示 Python 中运算符重载的代码示例
class Rectangle:
def __init__(self, length, width):
self.length = length
self.width = width
def __str__(self):
return f"Rectangle({self.length}, {self.width})"
def __add__(self, other):
return Rectangle(self.length + other.length, self.width + other.width)
r1 = Rectangle(4, 3)
r2 = Rectangle(2, 5)
print(r1 + r2) ### Output
在上面的代码中,我们为 Rectangle
类的“+”运算符定义了自定义行为。当我们使用“+”运算符添加两个 Rectangle
对象 时,它将创建一个新的 Rectangle
对象,其中包含两个矩形的 length
和 width
的总和。
class Book:
def __init__(self, title, author, pages):
self.title = title
self.author = author
self.pages = pages
def __lt__(self, other):
return self.pages < other.pages
b1 = Book("Python for Beginners", "John Smith", 300)
b2 = Book("Advanced Python", "David Johnson", 500)
print(b1 < b2) ### Output
在此代码中,我们为 Book
类定义了 <
运算符的自定义行为。它基于 pages
的数量比较两本书。如果 self
对象中的 pages
数量少于 other
对象中的 pages
数量,它将 return
True
。
总体而言,Python 中的运算符重载可以帮助您编写更具可读性和表现力的代码。它使您的代码看起来更简洁、更易于理解。
Python 中的数学运算符
Python 提供了各种数学运算符,可用于在 Python 中执行数学运算。这些运算符包括加法、减法、乘法、除法和模运算。
加法运算符
Python 中的加法运算符由 +
表示。它用于添加两个或更多个 数字,如下所示
# Adding two numbers
num1 = 10
num2 = 20
result = num1 + num2
print(result) # Output: 30
# Adding more than two numbers
result = num1 + num2 + 5
print(result) # Output: 35
除法运算符
Python 中的除法运算符由 /
表示。它用于将一个数字除以另一个数字,并返回一个浮点数作为结果
# Division of two numbers
num1 = 10
num2 = 2
result = num1 / num2
print(result) # Output: 5.0 (float)
# Division with remainder
num1 = 7
num2 = 3
result = num1 / num2
remainder = num1 % num2
print(result) # Output: 2.3333333333333335 (float)
print(remainder) # Output: 1 (int)
Python 提供了许多其他数学运算符,例如减法、乘法、模运算等。通过使用这些运算符,您可以在 Python 程序中执行复杂的数学运算。
Python 中的按位运算符
位运算符用于在 Python 中对整数执行按位运算。这些运算通过操作整数的二进制表示中的各个位来工作。这在低级编程中很有用,例如优化代码或使用硬件。
如何在 Python 中使用位运算符
Python 中有六个可用的按位运算符。这些是
-
&
(按位 AND):此运算符返回一个新整数,其位仅在操作数的两个相应位均为 1 时才设置为 1。 -
|
(按位 OR):此运算符返回一个新整数,其位在操作数的任何相应位为 1 时设置为 1。 -
^
(按位 XOR):此运算符返回一个新整数,其位仅在操作数的相应位之一为 1 时设置为 1。 -
~
(按位 NOT):此运算符返回操作数的补码,即它翻转操作数的所有位。 -
<<
(左移):此运算符将操作数的位向左移动指定数量的位。移动的位用 0 填充。 -
>>
(右移):此运算符将操作数的位向右移动指定数量的位。对于正数,移动的位用 0 填充,对于负数,用 1 填充。
&
运算符
x = 5
y = 3
z = x & y ### in binary
print(z) ### Output
在此示例中,我们使用按位 AND 运算符来查找 x
和 y
之间的公共位。结果为 1
,这是 x
和 y
中唯一设置为 1 的位。
<<
运算符
x = 7
y = 2
z = x << 1 ### in binary
print(z) ### Output
在此示例中,我们使用左移运算符将 x
的位向左移动一位。结果为 14
,这是二进制中的 1110
。左侧移动的位用 0
填充。
总体而言,Python 中的位运算对于优化代码和低级处理数字很有用。
运算符顺序
在 Python 中,就像在数学中一样,运算符的求值顺序是特定的。这称为运算顺序。Python 中的运算符优先级如下
- 括号
- 指数运算
- 乘法、除法和取模(从左到右)
- 加法和减法(从左到右)
在 Python 中编写表达式时,记住此顺序非常重要。
result = 5 + 3 * 2 # first 3*2 will be evaluated, then 5 will be added to the result
print(result) # output: 11
result = (5 + 3) * 2 # first 5+3 will be evaluated in the parentheses, then the result will be multiplied by 2
print(result) # output: 16
在第一个示例中,根据运算顺序,乘法运算在加法运算之前进行求值。在第二个示例中,根据运算顺序,括号内的加法运算首先进行求值。了解 Python 中的运算符顺序可以帮助避免错误并产生更准确的结果。
海象运算符
海象运算符是 Python 3.8 及更高版本中提供的一项新功能。它提供了一种简洁高效的方式,可在某些情况下将值分配给变量。
了解海象运算符
海象运算符表示为 := 符号,用于在单个表达式中将值分配给变量。它在重复调用 函数 或方法消耗大量资源的情况下特别有用,因为它可以帮助减少这些调用。
带有“if”语句的海象运算符
if (a:=2+3) > 4:
print(f"{a} is greater than 4")
else:
print(f"{a} is less than or equal to 4")
带有“while”循环的海象运算符
import random
while (num:=random.randint(1,10)) != 3:
print(num)
在以上两个示例中,海象运算符用于在与条件语句相同的表达式中将值分配给变量。这使得代码更简洁,更易于阅读。
指数运算符
Python 中的指数运算符表示为 **
,用于将数字乘方。它是一个二元运算符,需要两个操作数;第一个是底数,第二个是指数。
以下两个代码示例演示了在 Python 中使用指数运算符
num = 2
exp = 3
result = num ** exp
print(result)
num = 2
exp = 3
num **= exp
print(num)
在这两个示例中,**
运算符用于计算将数字乘方的 result
。在第二个示例中,result
使用赋值运算符 **=
分配给变量 num
,以获得更简洁的代码。通过使用指数运算符,您可以在 Python 代码中轻松执行指数运算。

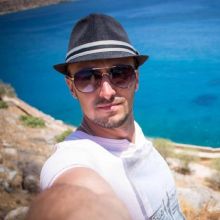