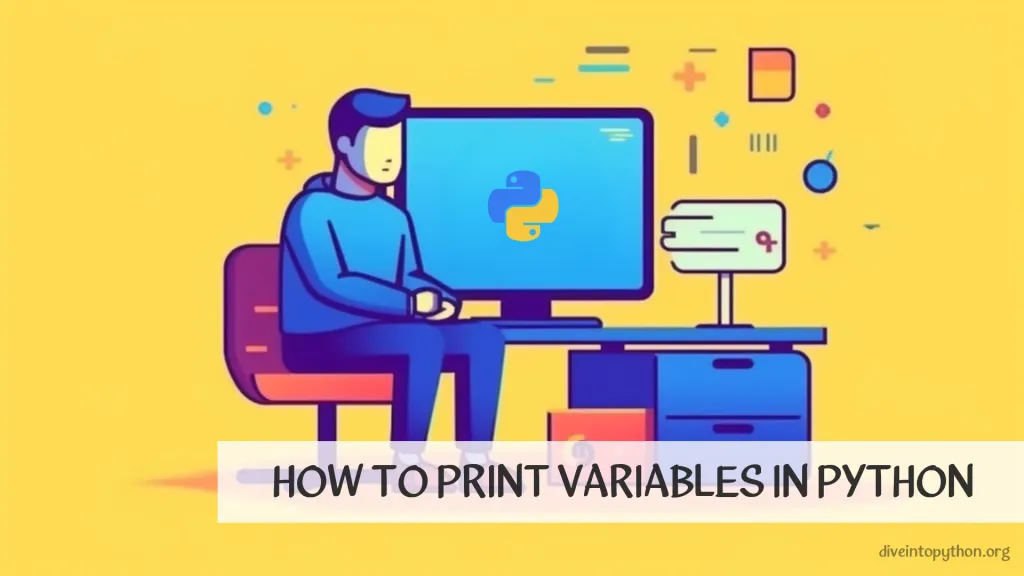
打印是指在控制台或终端上显示程序输出的过程。它是一项基本操作,允许你与用户通信或通过显示变量的值、计算结果或其他信息来调试代码。还有格式化这种操作,它通常用于打印。字符串格式化(也称为内插)是将变量或值插入字符串的过程。
打印
要打印 Python 中的变量,可以使用 print()
函数。
print()
函数接受一个或多个用逗号分隔的参数,并在控制台或终端上显示它们。
我们来看一些在 Python 中打印变量和字符串的示例
# Print a string
print("Hello, Python!")
# Print an integer
print(43)
# Print a floating-point number
print(3.14)
Python 还允许打印多个变量
# Print multiple items
print("The answer is:", 42)
你还可以对 print()
函数使用各种选项,例如指定项目之间的分隔符、以换行符结束输出以及将输出重定向到文件或其他流。
格式化
有多种方法可以格式化 Python 中的字符串,让我们逐一使用示例进行讲解。
- 使用 f 字符串:此方法涉及在字符串中使用占位符,然后用大括号
{}
中的值替换它们,大括号前加字母f
。例如
# Print using formatted strings
name = "Tom"
age = 32
print(f"My name is {name} and I am {age} years old.")
f 字符串
(或格式化字符串文字)是 Python 3.6 中引入的一项功能,它提供了一种使用以字母“f”开头的语法在字符串文字中嵌入表达式的便捷方法。
使用 f 字符串
,你可以通过将表达式括在大括号 {}
中来将它们嵌入字符串文字中。大括号中的表达式在运行时求值,并将它们的值插入字符串中。因此,这有助于在一个字符串中打印多个变量。
- 使用
format()
方法和大括号:你可以在字符串中使用{}
来指示要插入变量值的位置,然后使用format()
方法替换实际值。这是一个示例
name = "John"
age = 30
location = "New York"
print("My name is {}, I'm {} years old, and I live in {}.".format(name, age, location))
此代码将输出:我的名字是 John,我 30 岁,我住在纽约。
在此示例中,字符串 “我的名字是 {},我 {} 岁,我住在 {}。”
包含三个大括号 {}
,以指示应插入变量值的位置。对字符串调用 format()
方法,并将变量 name
、age
和 location
作为参数传递给该方法。
- 使用
%
运算符:此方法涉及在字符串中使用占位符,然后使用%
运算符替换它们。例如
name = "John"
age = 25
print("My name is %s and I'm %d years old." % (name, age)) # Output: My name is John and I'm 25 years old.
此处,%s
是字符串的占位符,%d
是整数的占位符。
字符串模板
字符串模板提供了一种创建字符串的方法,其中包含将稍后填入的值的占位符。当您想要基于某些输入生成动态字符串时,这会很有用。
要在 Python 中使用字符串模板,可以使用 string.Template
类。这是一个示例
from string import Template
name = "Alice"
age = 30
# Create a string template with placeholders for the name and age
template_str = "My name is ${name} and I am ${age} years old."
# Create a template object from the template string
template = Template(template_str)
# Substitute the values for the placeholders
result = template.substitute(name=name, age=age)
print(result) # Output: My name is Alice and I am 30 years old.
在上面的示例中,我们首先使用 ${}
语法为名称和年龄创建带占位符的字符串模板。然后,我们从模板字符串创建一个 Template
对象,并使用 substitute
方法用实际值替换占位符。最后,我们打印结果字符串。
请注意,您需要将占位符的值作为关键字参数传递给 substitute
方法。
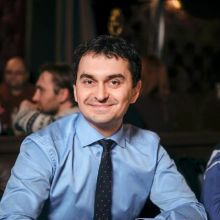

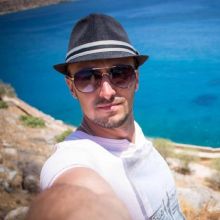