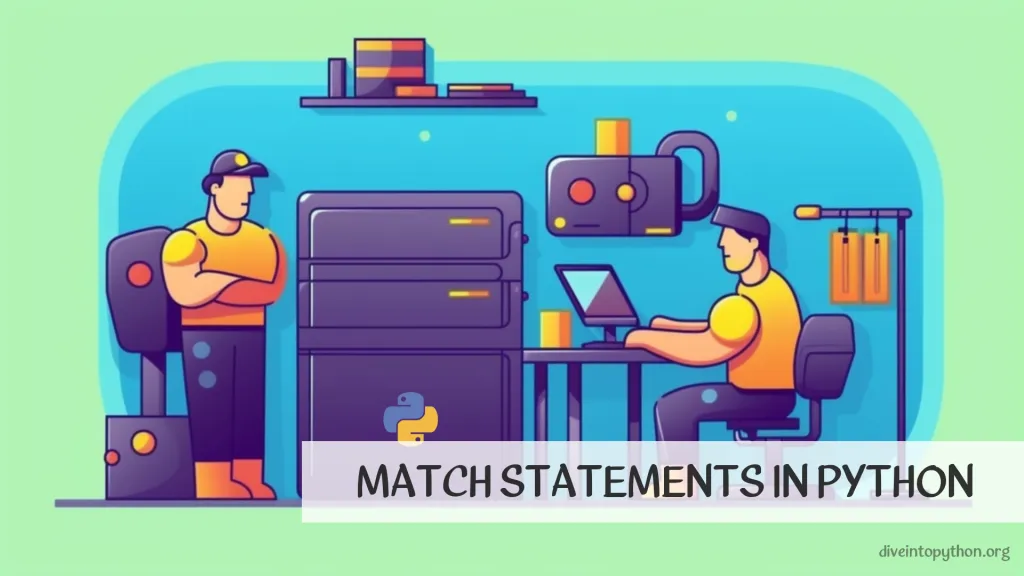
Switch 语句 是许多编程语言中流行的功能,它允许开发人员干净高效地处理多个条件情况。但是,Python 中没有内置的 switch 语句。在本文中,我们将探讨使用各种技术和库在 Python 中实现类似 switch 的功能的几种方法。无论您是经验丰富的开发人员还是刚入门,本指南都将为您提供对这一重要编程概念的宝贵见解。
匹配语句
match
语句在 Python 3.10 中引入,它提供了一种简洁易读的方式来表达条件逻辑。它允许您将一个值与一组模式进行比较,并根据匹配执行相应的代码。
要在 Python 中使用 match
,您可以为要匹配的每个模式创建一个 case
语句。以下是一个演示 Python 匹配 case 语句的示例代码片段
def describe_number(num):
match num:
case 0:
return "Zero"
case 1:
return "Single"
case _:
return "Multiple"
在此示例中,describe_number
函数采用一个 num
参数,并根据 num
的值返回一个字符串。如果 num
为 0
,则返回 Zero
。如果 num
为 1
,则返回 Single
。否则,它使用带有 _
的通配符匹配模式返回 Multiple
。
以下是如何使用 Python 编写 case 语句的另一个示例
def calculate_discount(total_amount):
match total_amount:
case amount if amount < 1000:
return amount * 0.05
case amount if amount >= 1000 and amount < 5000:
return amount * 0.10
case amount if amount >= 5000:
return amount * 0.15
在此示例中,calculate_discount
函数采用一个 total_amount
参数,并根据 total_amount
的值返回相应的折扣。match
语句有三个带有特定条件的 case
语句,每个语句都返回适用的折扣百分比。
总之,match
语句是 Python 语言的有力补充,它简化了条件语句。它可以提高可读性,并帮助您编写更简洁的代码。
Switch 语句
不幸的是,Python 没有本机 switch case 语句。但是,可以通过 if-elif-else 语句或 字典 等其他结构来模拟其功能。
def switch_case(argument):
if argument == 0:
return "Zero"
elif argument == 1:
return "One"
elif argument == 2:
return "Two"
else:
return "Invalid argument"
print(switch_case(2)) # Output: Two
def switch_case(argument):
return {
0: "Zero",
1: "One",
2: "Two"
}.get(argument, "Invalid argument")
print(switch_case(2)) # Output: Two
虽然不如 switch case 语句简洁,但这些替代方法可以在您的 Python 代码中提供类似的逻辑和可读性。

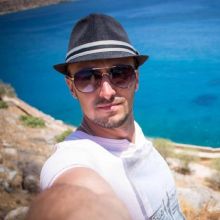