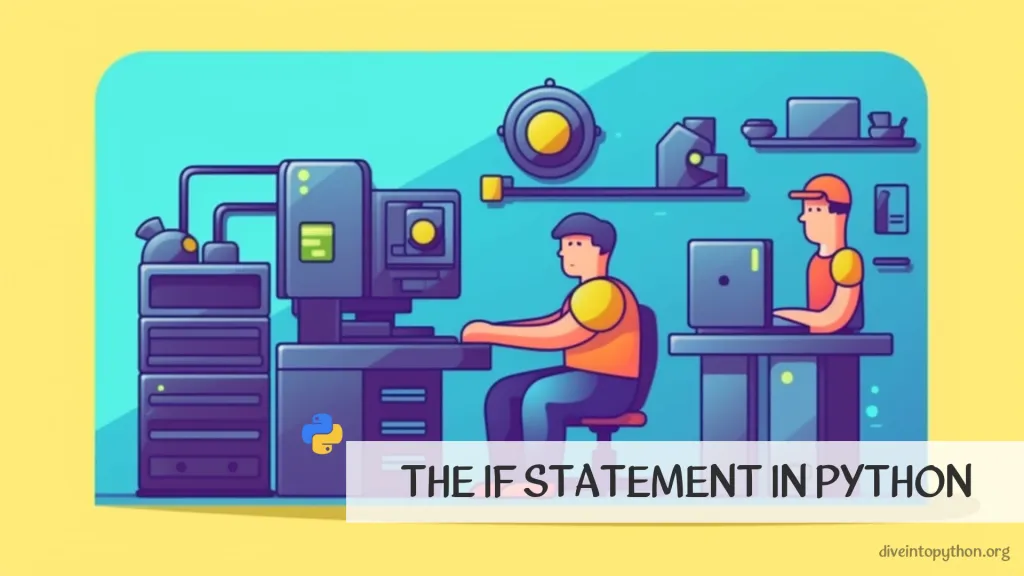
在 Python 中,if
语句用于有条件地执行代码。它允许你指定一个条件,并且仅当该条件计算结果为 True
时才执行代码块。
如何在 Python 中使用 if 语句
if
Python 中的语句 是基于特定条件执行代码块的条件语句。if
语句的基本语法为
if 1 > 2:
##### Execute block of code if the condition is true
pass
if 语句还可以与 else
语句结合使用,以基于 condition
为真或假执行不同的代码块。if-else 语句的语法为
if 2 > 1:
##### Execute block of code if the condition is true
print('True')
else:
### Execute block of code if the condition is false
print('False')
偶数
num = 4
if num % 2 == 0:
print("Even")
else:
print("Odd")
两个数字中的最大值
num1 = 10
num2 = 20
if num1 > num2:
print("num1 is greater than num2")
else:
print("num2 is greater than num1")
在 Python 中使用 if 语句来控制程序的流程,并基于特定条件做出决策。
三元运算符
三元语句提供了一种简洁的方法来编写单行 if 语句。它是一个内联 if 语句,包含三个操作数:一个条件、一个在条件为真时执行的表达式以及一个在条件为假时执行的表达式。
三元 if
语句语法
<expression_if_true> if <condition> else <expression_if_false>
检查一个数字是奇数还是偶数
num = 7
result = "Even" if num % 2 == 0 else "Odd"
print(result) # Output: Odd
在上面的示例中,三元语句使用 三元运算符 if
检查数字是奇数还是偶数。如果数字可以被 2 整除(即除法的余数为零),则 result
变量将被分配字符串 'Even'
,如果它不能被 2 整除(即余数为一),则 result
变量将被分配字符串 'Odd'
。
检查一个列表是否为空
my_list = [1, 2, 3]
result = "Not Empty" if my_list else "Empty"
print(result) # Output: Not Empty
在上面的示例中,三元语句检查 my_list
变量是否为空。如果 my_list
不为空,则 result
变量将被分配字符串 Not Empty
,如果它为空,则 result
变量将被分配字符串 Empty
。
总体而言,三元语句提供了一种简洁高效的方法来编写 Python 中的条件语句。
两个数字之间的 if 语句
要检查一个数字是否在 Python 中的两个 数字之间,你可以使用带有逻辑运算符的 if
语句。这里有两个示例
x = 5
if 2 <= x <= 8:
print("x is between 2 and 8")
else:
print("x is not between 2 and 8")
在这个示例中,if
语句检查 x
是否在 2
和 8
之间(包含)。如果 x
在 2
和 8
之间,则将执行语句 print(
x is between 2 and 8)
,否则,将执行 print(
x is not between 2 and 8)
。
y = 10
if not (y < 2 or y > 8):
print("y is between 2 and 8")
else:
print("y is not between 2 and 8")
在这个示例中,if
语句检查 y
是否不小于 2
或不大于 8
。如果 y
在 2
和 8
之间,则将执行语句 print(y is between 2 and 8)
,否则,将执行 print(y is not between 2 and 8)
。
使用这些示例检查您的数字是否介于 Python 中的两个数字之间。
列表解析中的 if 语句
在 Python 中,可以在列表解析中使用 if
语句来筛选仅满足特定条件的值。这对于创建仅包含所需值的新列表非常有用,而无需编写循环。
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9]
even_numbers = [num for num in numbers if num % 2 == 0]
print(even_numbers)
此代码创建一个名为 even_numbers
的新列表,其中仅包含原始列表 numbers
中的偶数。
words = ["apple", "banana", "cherry", "date"]
short_words = [word for word in words if len(word) < 6]
print(short_words)
此示例使用列表解析创建一个名为 short_words
的新列表,其中仅包含原始列表 words
中少于六个字符的单词。
通过在列表解析中使用 if
语句,我们可以大幅减少创建新筛选列表所需的代码量。这可以生成更具可读性和简洁性的代码,并且更易于维护。
嵌套 if 语句
当需要同时检查两个或更多条件时,在 Python 编程中使用嵌套 If 语句。它是在另一个条件语句内部的条件语句,创建了分层的决策结构。
age = 18
if age >= 18:
print("You are legally an adult")
if age == 18:
print("Congratulations on turning 18!")
else:
print("You are not yet an adult")
在此示例中,第一个 if
语句检查 age
是否大于或等于 18。如果为 True
,则第二个 if
语句检查 age
是否等于 18。如果两个条件都为 True
,则它会打印祝贺消息。如果不是,则它仅打印合法成人的消息。
x = 5
y = 10
z = 15
if x > y:
if x > z:
print("x is the largest")
else:
print("z is the largest")
else:
if y > z:
print("y is the largest")
else:
print("z is the largest")
在此示例中,有三个 变量,并且嵌套 if
语句检查其中哪一个是最大的。如果 x
大于 y
,则它检查 x
是否大于 z
。如果是 True
,则它打印 x is the largest
。如果第一个条件为 False
,则它检查 z
是否大于 y
。如果是 True
,则它打印 z is the largest
。如果这些条件都不为 True
,则 y
必须是最大的,并且它打印 y is the largest
。
这些只是简单的示例,用于说明 Python 中嵌套 If 语句的概念以及如何在编程中使用它们。
Python 中的 elif 语句
Python 中的 Elif statement
是一个条件 statement
,可帮助您检查程序中的多个条件。它与 if
和 else
语句结合使用。
语法
if condition:
statement(s)
elif condition:
statement(s)
else:
statement(s)
此处,condition
是一个布尔表达式,其结果为 True
或 False
。如果 condition
为 True
,则执行 if
块内的语句。如果 condition
为 False
,则执行 elif
块内的语句。如果任何条件都不为 True
,则执行 else
块内的语句。
age = 25
if age < 18:
print("You are a minor")
elif age >= 18 and age <= 65:
print("You are an adult")
else:
print("You are a senior citizen")
在此示例中,我们检查一个人的 age
。如果 age
小于 18,则打印 You are a minor
。如果 age
在 18 到 65 之间,则打印 You are an adult
。如果 age
大于 65,则打印 You are a senior citizen
。
score = 80
if score >= 90:
print("You have secured an A grade")
elif score >= 80 and score < 90:
print("You have secured a B grade")
elif score >= 70 and score < 80:
print("You have secured a C grade")
elif score >= 60 and score < 70:
print("You have secured a D grade")
else:
print("You have failed the exam")
在此示例中,我们检查一个学生的 score
。根据 score
,它打印不同的消息。如果 score
大于或等于 90,则打印 You have secured an A grade
。如果 score
在 80 到 89 之间,则打印 You have secured a B grade
。如果 score
在 70 到 79 之间,则打印 You have secured a C grade
。如果 score
在 60 到 69 之间,则打印 You have secured a D grade
。如果 score
小于 60,则打印 You have failed the exam
。
多个 if 语句
在 Python 中,可以使用多个 if
语句在单个执行块中检查多个条件。在 Python 中编写多个 if
语句有两种方法 - 使用多个 if
语句和使用多行 if
语句。
多个 if
语句的示例
使用多个 if
语句的语法如下
if condition1:
# do something
if condition2:
# do something else
if condition3:
# do something else again
此处,每个 if
语句都检查一个单独的条件,并在条件为 true 时执行相应的代码块。
x = 5
y = 8
if x > 0:
print("x is a positive number")
if y < 10:
print("y is less than 10")
# Output:
#
# x is a positive number
# y is less than 10
多行 if
语句的另一个示例
使用多行if
语句的语法如下
if condition1 and \
condition2 and \
condition3:
# do something
此处,反斜杠(\
)表示if
语句在下一行继续。
x = 5
y = 8
if x > 0 and \
y < 10:
print("x is a positive number and y is less than 10")
# Output:
#
# x is a positive number and y is less than 10
总体而言,这两种方法都可用于检查 Python 中的多个条件,但多行if
语句可以使代码更具可读性和易于管理。

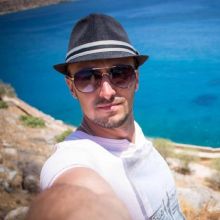