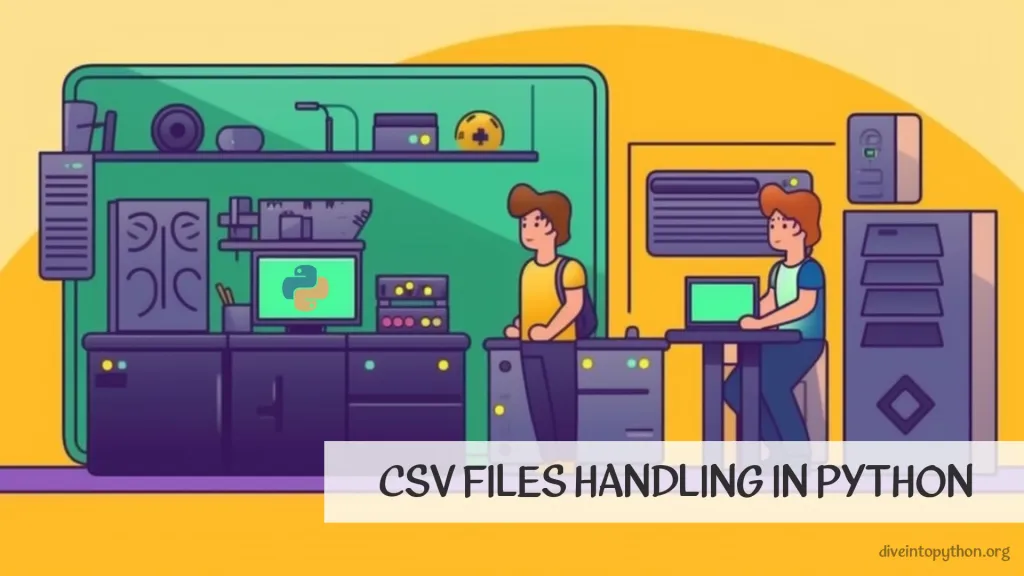
CSV(逗号分隔值)文件是数据科学、机器学习和分析中最常用的数据格式之一。Python 是一种功能强大的编程语言,它提供了多种工具和库来处理 CSV 文件。在本文中,我们将探讨在 Python 中处理 CSV 文件的基础知识,包括读取、写入和操作数据。我们还将介绍一些高级主题,例如处理大型 CSV 文件、处理缺失数据以及使用 NumPy 和 Pandas 库对 CSV 数据执行操作。
使用 Python 打开 CSV 文件并读取数据
要打开和读取 Python 中的 CSV 文件,可以使用内置的 csv 模块。
import csv
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
在此示例中,我们使用 csv.reader()
函数读取名为 example.csv
的 CSV 文件的内容。然后,我们使用 for
循环遍历文件中的行,并将每个 row
打印到控制台。
import csv
with open('example.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Age', 'Gender'])
writer.writerow(['John', '25', 'Male'])
writer.writerow(['Jane', '30', 'Female'])
在此示例中,我们使用 csv.writer()
函数将 data
写入名为 example.csv
的 CSV 文件。我们使用 w
模式创建新文件,并指定 newline=''
以避免额外的换行符。然后,我们使用 writerow()
函数将 data
的每一 row
写入文件。
通过使用这些代码示例,您可以轻松提供 CSV 读取 或 加载 CSV。
如何在 Python 中保存到 CSV 文件
在 Python 中保存数据到 CSV 文件是一项常见任务。CSV 文件易于读取,并且可以在任何电子表格软件中轻松打开。在 Python 中,我们可以使用 csv
模块写入 CSV 文件。以下是如何在 Python 中保存到 CSV 文件的一些示例。
此示例演示如何将简单的值列表写入 CSV 文件。
import csv
# Example data
data = [['Name', 'Age', 'Gender'], ['Alice', '25', 'Female'], ['Bob', '30', 'Male'], ['Charlie', '35', 'Male']]
# Open csv file in write mode
with open('example.csv', mode='w') as file:
writer = csv.writer(file)
# Write data to csv file
writer.writerows(data)
在上面的代码中
- 我们
import
csv
模块。 - 我们创建一个名为
data
的简单值列表。 - 我们使用
open()
函数以写入模式打开 CSV 文件,并将mode
指定为'w'
。 - 我们创建一个
csv.writer
对象并将文件对象传递给 writer。 - 我们使用
writerows()
方法将数据写入 CSV 文件。
此示例显示如何将值字典写入 CSV 文件。
import csv
# Example data
data = [{'Name': 'Alice', 'Age': '25', 'Gender': 'Female'},
{'Name': 'Bob', 'Age': '30', 'Gender': 'Male'},
{'Name': 'Charlie', 'Age': '35', 'Gender': 'Male'}]
# Open csv file in write mode
with open('example.csv', mode='w', newline='') as file:
fieldnames = ['Name', 'Age', 'Gender']
writer = csv.DictWriter(file, fieldnames=fieldnames)
writer.writeheader()
# Write data to csv file
for item in data:
writer.writerow(item)
在上面的代码中
- 我们
import
csv
模块。 - 我们创建一个名为
data
的字典列表。 - 我们使用
open()
函数以写入模式打开 CSV 文件,并将mode
指定为'w'
。我们还将newline
设置为''
以防止在每一行之间插入空行。 - 我们创建一个
csv.DictWriter
对象并将文件对象传递给writer
。我们还将fieldnames
提供为列表。 - 我们使用
writeheader()
方法将fieldnames
写入 CSV 文件。 - 我们使用
writerow()
方法将data
的每一row
写入 CSV 文件。
通过使用 Python 中的 csv
模块,你可以轻松地将数据保存到 CSV 文件中。这些示例可以根据你的特定要求进行修改。
如何使用 Python 将 JSON 转换为 CSV
将 json data
转换为 CSV 格式是 data
处理中的一项常见任务。Python 提供了一种使用内置 模块(例如 json
和 csv
)将 JSON data
转换为 CSV 格式的简单有效的方法。
使用 JSON 和 CSV 模块
import json
import csv
# Load JSON data
with open('data.json', 'r') as file:
data = json.load(file)
# Open CSV file for writing
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
# Write header row
writer.writerow(data[0].keys())
# Write data rows
for item in data:
writer.writerow(item.values())
使用 Pandas 库
import pandas as pd
# Load JSON data
with open('data.json', 'r') as file:
data = json.load(file)
# Convert to dataframe
df = pd.DataFrame(data)
# Write to CSV file
df.to_csv('data.csv', index=False)
在这两个示例中,我们从文件中加载 JSON 数据,将其转换为 Python 对象,然后使用 csv
模块或 pandas 库将其写入 CSV 文件。使用这些方法,你可以轻松地在 Python 中将 JSON 数据转换为 CSV 格式。
使用 Pandas 读取 CSV
Pandas 是一个功能强大的 Python 开源数据分析库,它为数据操作和分析提供了易于使用的数据结构。在 pandas
中,读取和操作 CSV 文件简单高效。
使用 Pandas 加载 CSV
要使用 Pandas 加载 CSV 文件,我们使用 read_csv()
。让我们看看如何使用 Pandas 加载 CSV 文件
import pandas as pd
df = pd.read_csv('filename.csv')
print(df.head())
使用 Pandas 解析 CSV 文件
加载 CSV 文件后,我们需要解析数据以提取所需信息。Pandas 提供了许多操作来解析和操作 CSV 数据。以下是如何使用 Pandas 解析数据的一个示例
import pandas as pd
df = pd.read_csv('filename.csv')
df = df[df['column_name'] == 'required_value']
print(df.head())
使用 Pandas 将 DataFrame 写入 CSV
处理 CSV 数据后,我们可能希望将新的 DataFrame 写入新的 CSV 文件。Pandas 提供了一种使用 to_csv()
将 DataFrame 写入 CSV 文件的简单方法。以下是一个示例
import pandas as pd
df = pd.read_csv('filename.csv')
# Perform operations to extract the required data
new_df = df[df['column_name'] == 'required_value']
# Write the new DataFrame to a new CSV file
new_df.to_csv('new_file.csv', index=False)
导出到 CSV
将 data
导出到 CSV(逗号分隔值)是 data
处理中的一项常见任务。以下是在 Python 中将 data
导出到 CSV 的两种方法
使用 csv 模块
csv 模块是 Python 中的一个内置模块,它支持 CSV 文件的读写。以下是如何使用 csv
模块将字典导出到 CSV 文件的一个示例
import csv
data = {'name': ['John', 'Jane', 'Adam'], 'age': [20, 25, 30]}
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(data.keys())
writer.writerows(zip(*data.values()))
# This code creates a CSV file with the following format:
#
#
# name,age
# John,20
# Jane,25
# Adam,30
使用 pandas 模块
以下是如何将 pandas DataFrame 导出到 CSV 文件的一个示例。
import pandas as pd
data = {'name': ['John', 'Jane', 'Adam'], 'age': [20, 25, 30]}
df = pd.DataFrame(data)
df.to_csv('data.csv', index=False)
此代码创建的 CSV 文件与上一个示例的格式相同。index=False
参数用于从 CSV 文件中删除默认的行索引列。
逐行读取 CSV
要逐行读取 Python 中的 CSV 文件,我们可以使用内置的 csv
。
逐行读取 CSV
import csv
with open('example.csv', newline='') as csvfile:
reader = csv.reader(csvfile)
for row in reader:
print(row)
在上面的示例中,我们打开 CSV 文件 example.csv
并将其分配给 csvfile
变量。然后我们创建一个 csv.reader
对象,我们可以使用 for
循环逐行迭代该对象。循环中的每个 row
都表示为一个值列表。
在 CSV 中写入新行
import csv
with open('example.csv', mode='a', newline='') as csvfile:
writer = csv.writer(csvfile)
row = ['value1', 'value2', 'value3']
writer.writerow(row)
在上面的示例中,我们在“追加”mode
中打开 CSV 文件 example.csv
并将其分配给 csvfile
变量。然后我们创建一个 csv.writer
对象,我们可以使用它通过 writerow()
方法向 CSV 文件写入新行。row
变量是要写入 CSV 文件中新行的值列表。
通过使用这些简单的示例,我们可以轻松地在 Python 中逐行读写 CSV 文件。
如何在 Python 中读取单列 CSV
要读取 Python 中的一列 CSV,你可以使用 csv.DictReader()
函数将 CSV 文件作为 字典 读取。以下有两个示例
import csv
with open('example.csv') as file:
reader = csv.DictReader(file)
for row in reader:
print(row['column_name'])
在此代码示例中,我们首先 import
csv
模块。然后我们使用 with
语句 打开 CSV 文件 example.csv
。我们使用 CSV 文件 file
创建一个名为 reader
的 DictReader
对象。然后我们遍历 reader
中的每个 row
并 print
每行中 column_name
的值。
import pandas as pd
data = pd.read_csv('example.csv')
column_data = data['column_name']
print(column_data)
在此代码示例中,我们首先 import
pandas
模块,并使用 read_csv()
函数和传递 CSV 文件名 example.csv
创建一个名为 data
的 DataFrame
。然后我们将 column_name
中的数据分配给一个新变量 column_data
。最后,我们 print
column_data
。

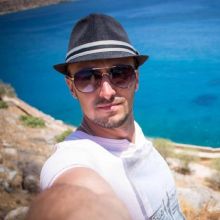