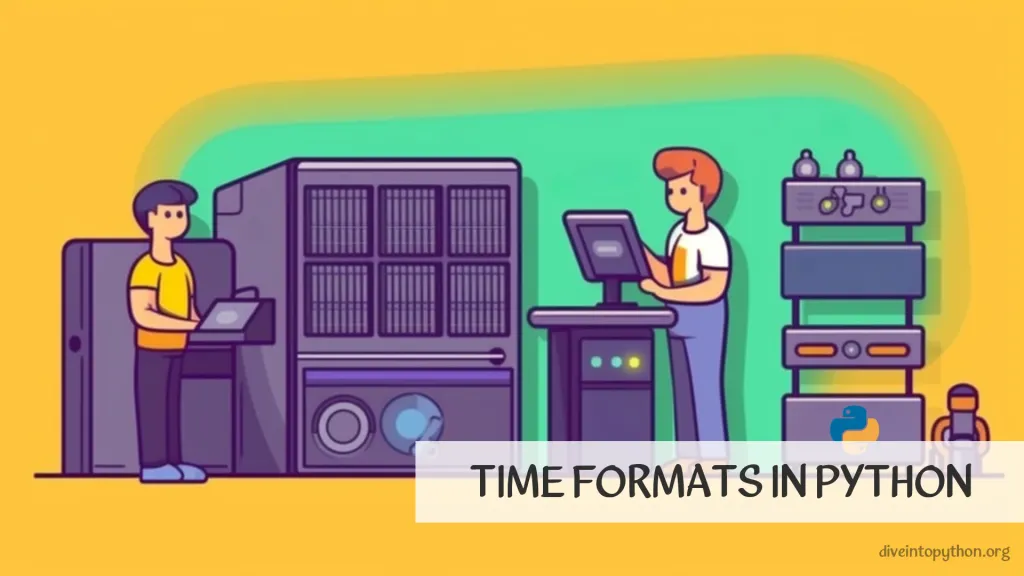
Python time 模块是用于处理 Python 中与时间相关的操作的强大工具。它提供用于测量时间间隔、格式化和解析时间和日期 字符串 以及处理时区的函数。借助 time 模块,你可以轻松处理时间和日期值,并在 Python 代码中执行各种与时间相关的操作。无论你需要测量代码的执行时间还是处理日期和时间值,Python 的 time 模块都能满足你的需求。
Python 中的时间格式
在 Python 中,你可以使用 time
模块中的 strftime()
方法根据各种格式代码格式化时间值。以下是 Python 中时间格式化中一些常用的格式代码
- %H:24 小时制中的 2 位小时(00-23)
- %I:12 小时制中的 2 位小时(01-12)
- %M:2 位分钟(00-59)
- %S:2 位秒(00-59)
- %p:AM/PM 标志(AM 或 PM)
你可以将这些格式代码与其他字符结合起来创建所需的时间格式。以下是如何在 Python 中获取格式化时间 的示例
import time
# Get the current time
current_time = time.localtime()
# Format the time using strftime()
formatted_time = time.strftime("%H:%M:%S", current_time)
formatted_time_am_pm = time.strftime("%I:%M:%S %p", current_time)
# Print the formatted time
print("Formatted Time (24-hour format):", formatted_time)
print("Formatted Time (12-hour format):", formatted_time_am_pm)
# Output:
# Formatted Time (24-hour format): 12:34:56
# Formatted Time (12-hour format): 12:34:56 PM
Python 的 time.sleep() 函数
time.sleep()
是一个 Python 函数,它挂起当前线程的执行指定秒数,因此你可以轻松设置自己的等待时间。
time.sleep()
的等待时间或延迟的语法如下
import time
time.sleep(seconds)
其中 seconds
是线程应该挂起的秒数。
sleep()
函数接受以秒为单位的睡眠持续时间,而不是毫秒。但是,你可以通过将所需的睡眠持续时间(以毫秒为单位)除以 1000 来实现类似的效果,将其转换为秒。
使用 time.sleep()
函数将帮助你暂停程序执行一段时间。
Python 中的 time.time() 函数
在 Python 中,time.time()
是一个函数,它返回自纪元(1970 年 1 月 1 日,00:00:00 UTC)以来当前时间的秒数,以浮点数形式表示。
以下是有关如何在 Python 中使用 time.time()
函数进行时间测量的示例
import time
start_time = time.time()
# do some time-consuming task here
end_time = time.time()
elapsed_time = end_time - start_time
print(f"The task took {elapsed_time:.2f} seconds to complete.")
在此示例中,start_time
和 end_time
是分别在耗时任务开始和结束时通过调用 time.time()
获得的。两个时间之间的差值给出经过的时间,然后将其打印出来。计算出的运行时间使用 f-string 格式化为带有两位小数的字符串。
这里还有使用 networkx
库在 Python 中进行图形创建的时间计算示例
import networkx as nx
import time
start_time = time.time()
# Create a graph with 100 nodes
G = nx.complete_graph(100)
end_time = time.time()
# Compute the time taken to create the graph
time_taken = end_time - start_time
print(f"Time taken to create the graph: {time_taken:.4f} seconds")
因此,time.time()
函数可用于在 Python 中计算时间。
以毫秒为单位测量时间
如果你想在 Python 中以毫秒为单位测量时间,可以使用 time
模块的 time()
函数以及 perf_counter()
函数。以下是一个示例
import time
# Get the current time in milliseconds
current_time_ms = int(time.time() * 1000)
print("Current Time in Milliseconds:", current_time_ms)
# Measure the execution time of a code block in milliseconds
start_time = time.perf_counter()
# Code or operation to measure
end_time = time.perf_counter()
execution_time_ms = int((end_time - start_time) * 1000)
print("Execution Time in Milliseconds:", execution_time_ms)
输出
Current Time in Milliseconds: 1621842353154
Execution Time in Milliseconds: 42
在此示例中,time.time()
用于检索当前时间,该时间以浮点数形式表示自纪元以来的秒数。通过将其乘以 1000,我们获得当前时间(以毫秒为单位)。
为了测量代码块的执行时间,我们使用 time.perf_counter()
以秒为单位获取当前的高分辨率时间。我们在代码块之前捕获开始时间,在代码块之后捕获结束时间。通过从结束时间中减去开始时间,我们获得经过的时间(以秒为单位)。将其乘以 1000,我们获得执行时间(以毫秒为单位)。
带示例的 Python timeit()
Python 的 timeit
模块是用于测量小代码片段执行时间的强大工具。它提供了一种简单的方法来对代码执行进行计时并比较不同方法的性能。当你想对不同的实现进行基准测试并确定哪一个更有效时,timeit
模块特别有用。
Python timeit()
参数
Python 中的 timeit
函数 采用几个参数,允许你自定义其行为
- stmt:这是你想测量的语句。它可以是包含单个语句或用分号分隔的多个语句的字符串。
- setup:此参数是可选的,用于设置要测量的代码的环境。它也可以是包含一个或多个语句的字符串。
- timer:此参数指定要使用的计时器函数。如果未指定,将使用当前平台的默认计时器。
- number:此参数确定代码执行的次数。执行次数越多,计时测量越准确。
在 Python 代码中对多行进行计时
你可以使用 timeit
模块对多行 Python 代码进行计时。以下是使用不同方法的两个示例。
示例 1:使用分号
import timeit
code_to_measure = """
def square_numbers():
result = []
for i in range(1000):
result.append(i ** 2)
return result
square_numbers()
"""
execution_time = timeit.timeit(stmt=code_to_measure, number=10000)
print(f"Execution time: {execution_time} seconds")
示例 2:使用三引号
import timeit
code_to_measure = '''
def square_numbers():
result = []
for i in range(1000):
result.append(i ** 2)
return result
square_numbers()
'''
execution_time = timeit.timeit(stmt=code_to_measure, number=10000)
print(f"Execution time: {execution_time} seconds")
在这两个示例中,我们定义了一个函数(square_numbers
),然后使用 timeit
模块调用它。要测量的代码用三引号括起来,使我们能够跨越多行。
timeit()
方法
timeit 模块提供了不同的方法来测量执行时间。以下是其中一些方法。
timeit.timeit()
timeit.timeit()
方法用于测量代码片段的执行时间。它将代码作为字符串、执行次数和一个可选的设置 语句。
import timeit
code_to_measure = "result = [i**2 for i in range(1000)]"
execution_time = timeit.timeit(stmt=code_to_measure, number=10000)
print(f"Execution time: {execution_time} seconds")
timeit.repeat()
timeit.repeat()
方法允许你重复测量多次,并返回执行时间列表。
import timeit
code_to_measure = "result = [i**2 for i in range(1000)]"
execution_times = timeit.repeat(stmt=code_to_measure, number=10000, repeat=5)
print(f"Execution times: {execution_times}")
在此示例中,代码执行了 10,000 次,测量重复了 5 次。
timeit.default_timer()
timeit.default_timer()
方法根据平台上可用的最高分辨率时钟返回当前时间。
import timeit
start_time = timeit.default_timer()
# Code to be measured
result = [i**2 for i in range(1000)]
end_time = timeit.default_timer()
execution_time = end_time - start_time
print(f"Execution time: {execution_time} seconds")
使用 timeit
方法,你可以选择最适合测量需求的方法,轻松比较不同代码实现的性能。
以毫秒为单位获取时间
要使用 Python 获取当前时间(以毫秒为单位),可以使用 time
模块及其 time()
函数,该函数返回自纪元(1970 年 1 月 1 日 00:00:00 UTC)以来的秒数。然后,你可以将此值乘以 1000 以获取毫秒为单位的时间
import time
milliseconds = int(round(time.time() * 1000))
print(milliseconds)
这将以毫秒为单位打印当前时间。请注意,在使用 int()
将其转换为整数之前,结果使用 round()
函数舍入为整数。这是因为 time.time()
返回一个具有高精度的浮点值。
Python 定时器
要在 Python 中创建计时器,可以使用内置的 time
模块。以下是一个示例代码片段,演示如何创建计时器
import time
def countdown(t):
while t:
mins, secs = divmod(t, 60)
timer = '{:02d}:{:02d}'.format(mins, secs)
print(timer, end="\r")
time.sleep(1)
t -= 1
print('Timer completed!')
# Set the time for the timer (in seconds)
t = 60
# Call the countdown function with the time value as the argument
countdown(t)
此代码将创建一个 60 秒倒计时器,并在计时器完成之前每秒在屏幕上打印剩余时间。你可以调整 t 的值以设置计时器的所需长度。
使用此功能,你可以创建一个计时器函数来测量经过的时间。
Python 中的时间函数
在 Python 中,time
模块提供了一系列用于处理时间的功能。以下是一些常用的函数(除了我们已经提到的 time()
和 sleep()
)
- ctime() - 此函数获取以秒为单位的时间,并返回该时间的字符串表示形式,格式为“Day Month Date Time Year”。示例用法
import time
current_time = time.time()
time_string = time.ctime(current_time)
print("Current time: ", time_string)
- gmtime() - 此函数获取以秒为单位的时间,并返回表示 UTC 时间的 struct_time 对象。示例用法
import time
current_time = time.time()
utc_time = time.gmtime(current_time)
print("UTC time: ", utc_time)
Python 的 time 模块中还有许多其他函数可用于处理时间,包括 localtime()
、strftime()
和 strptime()
。
时间模块中的 perf_counter() 函数
在 Python 中,time
模块中的 perf_counter()
函数用于测量系统上可用最高分辨率的经过时间。与常规 time()
函数相比,它提供了一个更精确的计时器。以下是如何使用 perf_counter()
的示例
import time
start_time = time.perf_counter()
# Code to be measured
for i in range(1000):
# Perform some computation
result = i ** 2
end_time = time.perf_counter()
execution_time = end_time - start_time
print(f"Execution time: {execution_time} seconds")
通过使用 perf_counter()
,你可以高精度地测量特定代码块的执行时间。此函数通常用于性能分析和基准测试目的。
时间模块的 monotonic() 函数
在 Python 中,time
模块中的 monotonic()
函数用于获取单调时钟,该时钟会持续增加且不受系统时间调整的影响。它适用于测量间隔和确定事件之间的经过时间。以下是如何使用 monotonic()
的示例
import time
start_time = time.monotonic()
# Code to be measured
for i in range(1000):
# Perform some computation
result = i ** 2
end_time = time.monotonic()
elapsed_time = end_time - start_time
print(f"Elapsed time: {elapsed_time} seconds")
使用 monotonic()
确保经过时间是根据单调时钟计算的,不受系统时间更改(例如时钟调整或时区更改)的影响。它为性能测量和基准测试目的提供了经过时间的可靠度量。
如何在一段时间后停止程序执行
如果你想在一段时间后停止 Python 程序的执行,可以使用 signal
模块和计时器。signal
模块允许你处理各种信号,包括计时器信号,以控制程序的行为。以下是一个示例,演示如何在指定持续时间后停止程序
import signal
import time
# Define the handler function for the alarm signal
def timeout_handler(signum, frame):
raise TimeoutError("Program execution timed out")
# Set the duration (in seconds) after which the program should be stopped
duration = 10
# Register the handler function to be called when the alarm signal is received
signal.signal(signal.SIGALRM, timeout_handler)
# Set the alarm to trigger after the specified duration
signal.alarm(duration)
try:
# Code to be executed
while True:
# Perform some computation
time.sleep(1) # Simulate some work
except TimeoutError:
print("Program execution stopped after the specified duration")
在此示例中,程序使用 signal.alarm(duration)
设置警报,其中 duration 是以秒为单位的所需持续时间。当警报在指定持续时间后触发时,它会引发 TimeoutError
异常,该异常会被 try-except 块捕获。在 except 块中,你可以处理程序终止或打印消息以指示程序已停止。
注意:signal 模块并非在所有平台上都可用,其行为可能有所不同。此外,此方法可能不适用于中断长时间运行或计算密集型任务。对于更复杂的情况,你可能需要考虑多处理或线程技术来实现所需的行为。
如何在 Python 中测量函数的执行时间
要对 Python 中的特定函数的执行进行计时,可以使用 time
模块和装饰器。以下是如何使用装饰器对函数进行计时
import time
def timer_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
elapsed_time = end_time - start_time
print("Elapsed Time: {:.6f} seconds".format(elapsed_time))
return result
return wrapper
# Example function to be timed
@timer_decorator
def my_function():
time.sleep(2) # Simulating some time-consuming operation
return "Finished"
# Call the function
my_function() # Output: Elapsed Time: 2.001987 seconds
在此示例中,timer_decorator
函数是一个装饰器,它使用计时功能包装目标函数(my_function
)。装饰器在调用函数之前记录开始时间,在函数完成后记录结束时间。它计算经过时间并打印出来。
将 @timer_decorator
装饰器应用于 my_function
后,在调用该函数时会自动对其进行计时。
可以通过在函数定义上方应用 @timer_decorator
装饰器,将该装饰器用于要计时的任何函数。
注意:该示例使用
time.sleep(2)
函数调用来模拟耗时操作。请将其替换为您要计时的实际代码或操作。
如何获取当前时间
如果您想知道现在是什么时间,可以使用 time
模块的 time()
函数。以下是获取 Python 中当前时间的示例
import time
current_time = time.time()
print("Current Time (in seconds since the epoch):", current_time)
在此示例中,time.time() 将当前时间作为浮点数返回,表示自纪元以来的秒数。因此,我们可以通过这种方式获取 Python 中的当前 Unix 时间。
time.strftime() 函数
Python 的 time
模块中的 strftime()
函数用于根据指定的格式代码将 time.struct_time
对象或时间元组格式化为字符串表示形式。以下是一个示例
import time
# Get the current time as a time tuple
current_time = time.localtime()
# Format the time using strftime()
formatted_time = time.strftime("%Y-%m-%d %H:%M:%S", current_time)
# Print the formatted time
print("Formatted Time:", formatted_time) # Output: Formatted Time: 2023-05-22 12:34:56
在 Python 中打印时间
要打印 Python 中的当前时间,可以使用 time
模块或 datetime
模块。以下是如何使用 time
模块的示例
import time
current_time = time.localtime()
formatted_time = time.strftime("%H:%M:%S", current_time)
print("Current Time (using time module):", formatted_time)
如何获取当前时间
要检索 Python 中的当前时间,可以使用 time
模块或 datetime
模块。以下是如何使用 time
模块获取当前时间的示例
import time
current_time = time.localtime()
print("Current Time (using time module):", time.strftime("%H:%M:%S", current_time))
以下是如何获取当前时间的毫秒数的示例
import time
current_time_ms = int(time.time() * 1000)
print("Current Time (in milliseconds using time module):", current_time_ms)
在 Python 中生成时间戳
可以使用 time
模块生成时间戳。以下是一个示例
import time
timestamp = int(time.time())
print("Timestamp (using time module):", timestamp)
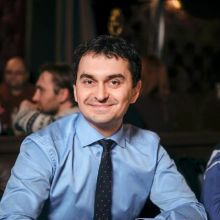

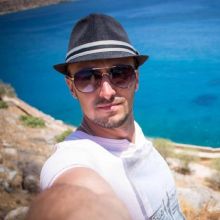