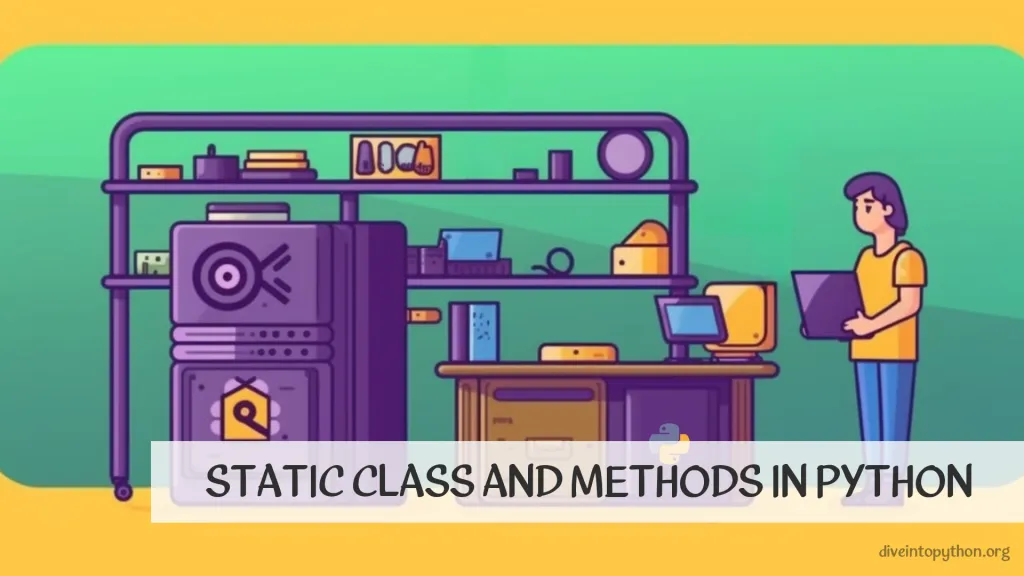
Python 提供了多种类类型,包括 Python 静态类,它允许你在无需创建实例的情况下定义类级别功能。使用静态类的一个优点是它允许你将相关的 函数 分组在一个类中,通过将 Python 静态类集成到你的 Python 项目中,你可以利用有组织的、不依赖于类实例的类级别功能的优势。要在类中创建静态方法,你可以使用 @staticmethod
装饰器。创建后,你可以直接从类调用静态方法,而无需创建它的实例。在本文中,我们将探讨静态类的概念,以及如何在类中创建和调用静态方法。
了解 Python 静态类
Python 静态类是一个不需要创建实例的类。它使用 @staticmethod
装饰器在类定义中创建。静态方法无法访问实例,它也不能修改类本身。
如何在 Python 静态类中使用和调用静态方法
除了理解静态方法的概念之外,掌握 Python 中静态类的重要性至关重要。
class MyClass:
@staticmethod
def static_method():
print("This is a static method in a class")
MyClass.static_method() # Output: This is a static method in a class
在上面的示例中,static_method()
是 MyClass
中的静态方法。它可以使用类名调用,而无需类的实例。
class Calculator:
@staticmethod
def add_numbers(x, y):
return x + y
result = Calculator.add_numbers(3, 5)
print(result) # Output: 8
在上面的示例中,Calculator
类的 add_numbers()
静态方法可以使用类名 Calculator
直接调用。它接受两个参数 x
和 y
,并返回它们的和。
总体而言,静态类和静态方法提供了一种表示不依赖于任何实例或类变量的功能的方法,并且可以在不创建类实例的情况下使用。
什么是 Python 中的静态方法
Python 允许我们在类中创建静态类和静态方法。Python 中的静态方法不需要在使用前创建类的实例。同时,Python 类静态方法是一种其第一个参数是类本身而不是类实例的方法。
要创建静态类和静态方法,我们只需在 Python 中使用 @staticmethod
装饰器。
class Math:
@staticmethod
def add(x, y):
return x + y
@staticmethod
def subtract(x, y):
return x - y
# To call the static methods in the class, we don't need to create an instance of the class
print(Math.add(2, 3)) # Output: 5
print(Math.subtract(5, 2)) # Output: 3
在上面的示例中,我们创建了一个名为 Math 的静态类,其中包含两个名为 add 和 subtract 的静态方法。我们可以直接从类调用这些方法,而无需创建类的实例。
class Person:
count = 0
def __init__(self, name):
self.name = name
Person.count += 1
@staticmethod
def total_people():
return f"There are {Person.count} people."
# Create some instances of Person
person1 = Person("John")
person2 = Person("Jane")
person3 = Person("Jack")
# Call the static method from the class
print(Person.total_people()) # Output: There are 3 people.
在此示例中,我们创建了一个 Person
类,其中包含一个名为 count
的类变量,它将跟踪创建的类实例的数量。我们还创建了一个名为 total_people
的Python 类静态方法,它将返回创建的总人数。我们可以直接从类调用此静态方法。
如何在类中调用静态方法
要调用类中的静态方法,你需要使用内置装饰器 @staticmethod
定义一个静态方法。静态方法不需要任何实例就能被调用,而是与类本身关联。
class MyClass:
@staticmethod ### Defining static method
def my_static_method():
print("This is a static method.")
### Calling the static method using the class name
MyClass.my_static_method() ### Output
class Math:
@staticmethod ### Defining static method
def add(a, b):
return a + b
@staticmethod ### Defining static method
def multiply(a, b):
return a * b
### Calling the static methods in class using class name
print(Math.add(2, 3)) ### Output 5
print(Math.multiply(2, 3)) ### Output 6
在上面的示例中,我们可以看到如何使用 Python 中的 @staticmethod
装饰器定义和调用静态方法。
何时使用静态方法
Python 中的静态方法是一个强大的特性,可以显著提高代码组织和可维护性。但是,了解何时何地有效地使用它们至关重要。以下是一些静态方法可能会有益的常见场景
实用函数
静态方法非常适合不与类的特定实例绑定的实用函数。这些函数提供可以在代码库的各个部分使用的功能。通过将它们封装在静态方法中,你可以确保一个干净且模块化的结构,使你的代码更易于维护。
class StringUtils:
@staticmethod
def reverse_string(string):
return string[::-1]
在此示例中,reverse_string
方法是一个实用函数,可用于反转字符串。它不需要访问特定于实例的数据,并且可以直接在类上调用。
工厂方法
静态方法可用于创建类的实例。当你想在类本身中封装对象创建逻辑时,这尤其有用。工厂方法简化了对象初始化的过程,并为创建 对象 提供了清晰简洁的接口。
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
@staticmethod
def create_square(side_length):
return Rectangle(side_length, side_length)
在此示例中,create_square
静态方法是一个工厂方法,它简化了正方形 Rectangle
实例的创建。
缓存和备忘
静态方法可用于缓存或备忘目的。当你需要存储和重用计算结果时,静态方法可以帮助在类中维护一个缓存,从而使后续计算更有效率。
class MathUtils:
_fib_cache = {0: 0, 1: 1}
@staticmethod
def fibonacci(n):
if n not in MathUtils._fib_cache:
MathUtils._fib_cache[n] = MathUtils.fibonacci(n - 1) + MathUtils.fibonacci(n - 2)
return MathUtils._fib_cache[n]
在此示例中,fibonacci
静态方法计算斐波那契 数字,并使用备忘来优化性能。
代码组织
使用静态方法将相关函数分组在一个类中。这可以改善代码组织和可读性。当函数共享一个共同的目的,但并不依赖于特定于实例的数据时,将它们封装为同一类中的静态方法可以使你的代码库保持结构化。
class FileUtils:
@staticmethod
def read_file(filename):
# Read file content
@staticmethod
def write_file(filename, content):
# Write content to a file
在此示例中,FileUtils
类将与文件操作相关的函数分组在一起,提供了一种清晰且有组织的方式来处理文件。
通过识别这些场景,你可以有效地利用 Python 代码库中静态方法的力量,并创建更易于维护和组织的软件。静态方法提供了一种干净简洁的方式来封装不依赖于特定于实例的数据的功能,同时提高代码的可读性和可维护性。
静态类与常规类
Python 中的静态 类 是具有类级属性和方法的类,可以在不创建类实例的情况下访问这些属性和方法。它们使用 @staticmethod
装饰器定义。另一方面,Python 中的常规类是需要类的对象才能访问属性或方法的类。
Python 静态类
class StaticClass:
@staticmethod
def my_static_method():
print("This is a static method")
# Call static method in class
StaticClass.my_static_method()
在上面的示例中,我们定义了一个静态类 StaticClass
,其中有一个静态方法 my_static_method
。我们可以在不创建类实例的情况下调用此方法。
如何在 Python 中使用静态类
Python 中的静态类是用于对无法归类到现有类下的函数进行分组的类。这些类不需要创建类的实例即可被访问。相反,静态方法可以直接从类本身调用。以下是使用静态类的一些主要好处
-
命名空间组织 - 静态类可用于组织不适合任何现有类的函数。这有助于维护一个干净且有组织的代码结构。
-
代码可重用性 - 静态类可以在多个 模块 和函数中重用,使代码可重用且易于维护。
-
提高代码可读性 - 静态类使代码更容易阅读和理解,因为函数被分组在一个类下,并且可以直接访问。
class MathUtils:
@staticmethod
def add_numbers(a, b):
return a + b
result = MathUtils.add_numbers(2, 3)
print(result)
在此示例中,我们创建了一个静态类 MathUtils
,并定义了一个静态方法 add_numbers
,它接受两个参数并返回它们的和。静态方法可以直接从类 MathUtils
调用,而无需创建类的实例。
class StringUtils:
@staticmethod
def reverse_string(string):
return string[::-1]
class TextUtils:
@staticmethod
def reverse_and_uppercase(string):
reversed_string = StringUtils.reverse_string(string)
return reversed_string.upper()
result = TextUtils.reverse_and_uppercase("Hello World")
print(result)
在此示例中,我们创建了两个静态类 StringUtils
和 TextUtils
。StringUtils
类包含一个静态方法 reverse_string
,它返回一个反转的字符串。TextUtils
类包含一个静态方法 reverse_and_uppercase
,它从 StringUtils
类调用 reverse_string
方法,并返回大写的反转字符串。
类中的静态变量
在 Python 中,静态变量是类级变量,在类的所有实例之间共享。这些变量在类中定义,但在任何方法之外,并且它们在类的不同实例之间保留其值。
当你想存储由类的所有实例共享的数据,或者当你想在所有实例之间维护一个计数或一个公共配置时,静态变量很有用。
以下是两个代码示例,演示了在 Python 类中使用静态 变量 的用法
示例 1:计数实例
class Car:
# Static variable to keep track of the number of instances
count = 0
def __init__(self, name):
self.name = name
# Increment the count when a new instance is created
Car.count += 1
# Creating instances of the Car class
car1 = Car("Tesla")
car2 = Car("BMW")
car3 = Car("Audi")
# Accessing the static variable using the class name
print("Total cars:", Car.count) # Output: Total cars: 3
在上面的示例中,count
变量是一个静态变量,用于跟踪创建的 Car
实例的数量。每当创建新实例时,它都会在构造函数 (__init__
) 中递增。使用类名 (Car.count
) 访问静态变量。
示例 2:通用配置
class Circle:
# Class-level constant for pi
PI = 3.14159
def __init__(self, radius):
self.radius = radius
def calculate_area(self):
# Accessing the static variable inside an instance method
area = Circle.PI * self.radius * self.radius
return area
# Creating instances of the Circle class
circle1 = Circle(5)
circle2 = Circle(7)
# Calling the instance method to calculate the area
area1 = circle1.calculate_area()
area2 = circle2.calculate_area()
print("Area 1:", area1) # Output: Area 1: 78.53975
print("Area 2:", area2) # Output: Area 2: 153.93791
在此示例中,PI
变量是一个静态变量,用于存储 pi
的值。它在 calculate_area 方法中用于计算圆的面积。使用类名 (Circle.PI
) 访问静态变量。
静态变量在类的所有实例之间共享,可以使用类名或通过类的实例访问。它们是管理所有实例共用的数据或维护对象之间的共享配置的有用工具。
类方法和静态方法之间的区别
现在你已经熟悉了类方法和静态方法,是时候深入了解 Python 的静态类及其独特属性了。
抽象类方法被定义为已声明但没有包含任何实现的方法。由子类提供实现。在 Python 中,使用 @abstractmethod 装饰器定义抽象方法。
类方法用于修改类或其属性。它们使用 @classmethod
装饰器定义,并将类作为其第一个参数传递,而不是实例。
Python 静态方法在类上调用,不接受任何特殊的第一参数。它们使用 @staticmethod
装饰器定义。
Python 中类方法与静态方法之间的主要区别
类方法 | 静态方法 |
---|---|
装饰器:@classmethod | 装饰器:@staticmethod |
第一个参数:cls(类本身) | 没有特殊的第一参数 |
可以修改类状态或属性 | 不能修改类状态或属性 |
适用于特定于类的操作 | 适用于与类无关的实用操作 |
可以访问类级变量 | 不能访问类级变量 |
示例 1:类方法
class Car:
wheels = 4
def __init__(self, make, model):
self.make = make
self.model = model
@classmethod
def set_wheels(cls, num_wheels):
cls.wheels = num_wheels
car1 = Car('Toyota', 'Corolla')
print(car1.wheels) # Output: 4
Car.set_wheels(3)
print(car1.wheels) # Output: 3
示例 2:静态方法
class Formatter:
@staticmethod
def format_string(string):
return string.upper()
print(Formatter.format_string('hello')) # Output: 'HELLO'

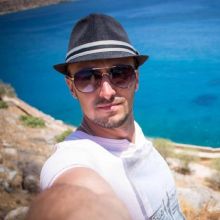