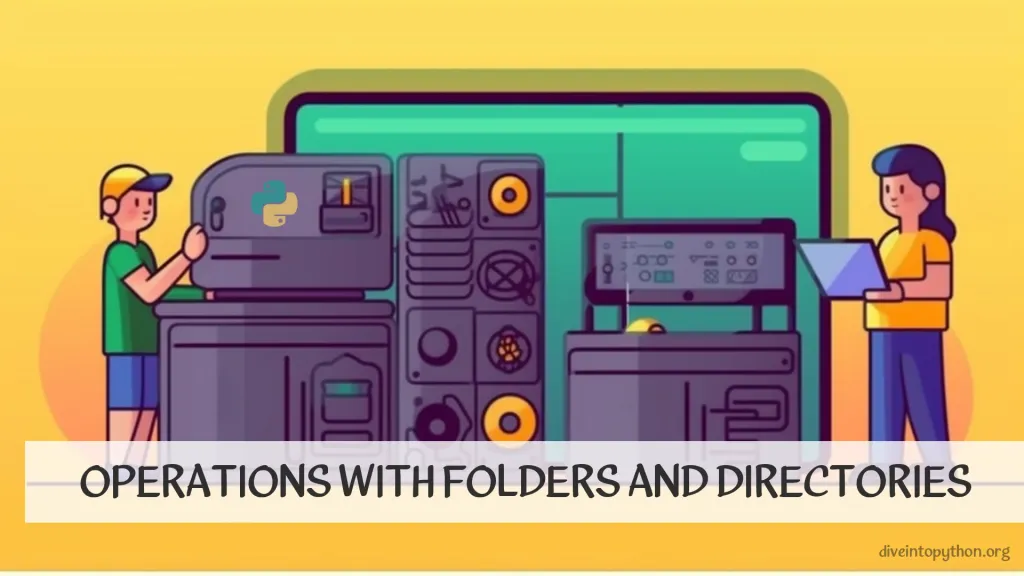
目录是文件管理的关键部分,在 Python 中开发全面程序时发挥着至关重要的作用。
OS 模块
Python 中的 os 模块提供了一种使用操作系统相关功能的方法。它有助于执行各种操作,例如创建目录、删除文件
、更改当前工作目录
等。
要使用 OS 模块,首先我们需要使用import os
语句导入
它。以下是使用 OS 模块的两个示例
示例 1:创建新目录
要使用 OS 模块创建目录,我们可以使用os.mkdir()
函数。这是一个示例
import os
# specify the path where you want to create the directory
path = "/home/user/newdir"
# create the directory
try:
os.mkdir(path)
print("Directory created successfully!")
except OSError as error:
print(error)
示例 2:获取当前工作目录
要使用 Python 中的os
模块获取当前工作目录,我们可以使用os.getcwd()
函数。这是一个示例
import os
# get the current working directory
cwd = os.getcwd()
# print the current working directory
print("Current working directory:", cwd)
通过使用os
模块,我们可以执行与操作系统相关的各种操作,包括文件操作、目录
操作和进程管理。
如何获取目录中的文件列表
要获取目录中的文件列表,可以使用os.listdir()
函数。此函数返回指定目录中所有文件和目录的列表。
import os
# get list of files in the current directory
files = os.listdir()
# print the list of files
print(files)
### Output
# ['file1.txt', 'file2.jpg', 'directory1', 'directory2']
import os
# get list of files in a directory
directory = "/path/to/directory"
files = os.listdir(directory)
# print the list of files
print(files)
### Output
# ['file1.pdf', 'file2.docx', 'file3.txt']
总之,通过使用os.listdir()
,你可以轻松获取目录中的文件列表。这对于各种任务很有用,例如文件操作、数据分析等。
如何获取当前目录
要获取当前目录,可以使用os
模块。os.getcwd()
方法将当前工作目录作为字符串返回。
import os
### Get current directory
current_directory = os.getcwd()
### Print current directory
print(current_directory)
你还可以使用 pathlib 模块获取当前目录
from pathlib import Path
### Get current directory
current_directory = Path.cwd()
### Print current directory
print(current_directory)
要更改当前目录,可以使用os.chdir()
方法
import os
### Change current directory
os.chdir('/path/to/new/directory')
### Get current directory
current_directory = os.getcwd()
### Print current directory
print(current_directory)
在尝试更改当前工作目录之前,请记住导入
用于更改目录的必需模块。
从另一个目录导入
要从另一个目录导入
模块,可以使用以下代码
import sys
sys.path.insert(0, '../path/to/parent/directory')
from module_name import function_name
在上面的示例中,我们首先使用sys.path.insert
将路径
插入到系统路径
中。接下来,我们可以使用from
关键字从指定的目录
中导入
所需的模块或函数。
从父目录导入
模块的另一种方法是使用__init__.py
文件。当目录作为模块导入时,此文件是一个特殊的可执行文件。在此文件中,你可以添加以下代码
import os
import sys
sys.path.append(os.path.dirname(os.path.abspath(__file__)) + "/../")
from module_name import function_name
在此方法中,我们首先import
os
模块以访问文件路径和目录信息。接下来,使用 sys.path.append
将父目录添加到系统path
。最后,我们可以使用 from
关键字从父目录import
所需的模块或函数。
通过使用这些方法,您可以轻松地从父目录import
模块并简化项目的组织。
检查目录是否存在
要检查目录是否存在,可以使用 os.path.exists()
函数。如果目录存在,它将返回 True
,否则将返回 False
。
import os
if os.path.exists('/my/directory'):
print('Directory exists')
else:
print('Directory does not exist')
如果目录不存在,您可以使用 os.makedirs()
函数创建目录。这将创建目录和所有必要的父目录。
import os
if not os.path.exists('/my/directory'):
os.makedirs('/my/directory')
### Now you can use the directory for your operations
确保将 /my/directory
替换为要检查或创建的目录的实际路径。
在 Python 中创建目录
要在 Python 中创建目录,可以使用 os
模块。您还可以使用 os.path.exists()
方法检查目录是否存在,然后再创建目录。如果 os.makedirs()
方法不存在,它将创建目录及其所有父目录。
import os
# Directory Path
path = "/example/directory"
# Check if directory already exists
if not os.path.exists(path):
# Create directory
os.makedirs(path)
print("Directory created successfully")
else:
print("Directory already exists")
os.mkdir()
方法仅在目录不存在时才创建目录。如果目录已存在,它将引发 FileExistsError
。
import os
# Directory Path
path = "/example/directory"
try:
# Create directory
os.mkdir(path)
print("Directory created successfully")
except FileExistsError:
print("Directory already exists")
使用其中任何一种方法,您都可以在 Python 中轻松创建目录并检查它是否存在。
将文件复制到另一个目录
在 Python 中将文件从一个目录复制到另一个目录是一项常见任务。有几种方法可以实现此目的,但最简单的方法是使用 shutil 模块,该模块提供了一个 copy
函数来复制文件。
使用 shutil.copy()
将文件从一个目录复制到另一个目录
shutil
模块中的 copy
函数有两个参数 - 源文件和目标目录。
import shutil
src_file = '/home/user/filename.txt'
dst_dir = '/home/user/new_directory'
shutil.copy(src_file, dst_dir)
这将把文件 filename.txt
从目录 /home/user
复制到目录 /home/user/new_directory
。
使用 os.rename()
在 Python 中将文件从一个目录移动到另一个目录
在 Python 中将文件从一个目录复制到另一个目录的另一种方法是使用 os.rename()
函数。
import os
src_file = '/home/user/filename.txt'
dst_dir = '/home/user/new_directory'
new_path = os.path.join(dst_dir, os.path.basename(src_file))
os.rename(src_file, new_path)
这将把文件 filename.txt
从目录 /home/user
移动到目录 /home/user/new_directory
。
总之,以上两种方法可以轻松地在 Python 中将文件从一个目录复制到另一个目录。
如何删除目录
要在 Python 中删除目录,我们可以使用 os
模块,该模块为我们提供了删除文件和目录的必要方法。删除目录中所有文件的一种方法是遍历所有文件并逐个删除它们。另一种方法是使用 os.rmdir()
方法简单地删除目录。
删除目录中的所有文件
我们可以通过遍历每个文件并使用 os.remove()
方法删除它们来删除目录中的所有文件。这是一个例子
import os
dir_name = '/path/to/directory'
# iterate over each file and delete it
for file_name in os.listdir(dir_name):
file_path = os.path.join(dir_name, file_name)
if os.path.isfile(file_path):
os.remove(file_path)
删除目录
我们可以使用 os.rmdir()
方法删除目录。此方法仅在目录为空时才有效 - 如果目录中存在任何文件,该方法将引发错误。要删除非空目录,我们可以使用 shutil
模块的 rmtree()
方法。这是一个例子
import os
import shutil
dir_name = '/path/to/directory'
# remove the directory
try:
os.rmdir(dir_name)
except OSError:
shutil.rmtree(dir_name)
使用上述代码示例,您现在可以使用 Python 轻松删除目录中的所有文件并删除目录本身。
读取目录中的所有文件
如果您想使用 Python 读取目录中的所有文件
,可以使用 os
模块。以下是如何完成此任务的两个示例
import os
### Method 1
files = os.listdir('/path/to/directory')
for filename in files:
with open(filename, 'r') as f:
##### do something with the file
pass
### Method 2
for dirpath, dirnames, filenames in os.walk('/path/to/directory'):
for filename in filenames:
with open(os.path.join(dirpath, filename), 'r') as f:
##### do something with the file
pass
在方法 1 中,os.listdir()
函数返回目录中所有 filenames
的列表。然后,你可以使用 for
循环遍历列表并打开每个文件。
在方法 2 中,os.walk()
函数遍历目录树,为其找到的每个目录返回 (dirpath,
dirnames, filenames)
的 3 元组。然后,你可以使用嵌套 for
循环遍历所有 filenames
并打开每个文件。
在这两种情况下,你可能需要调整路径 /path/to/directory
以匹配要读取的目录的实际位置。
如何从父目录导入
要在 Python 中从父目录 import
模块,你需要将父目录添加到系统的 sys.path
列表中。这可以使用 sys.path.append()
函数完成。
- 如果你的工作目录是父目录的子目录,则可以像这样将父目录添加到路径中
import sys
sys.path.append('..')
# from parent_module import parent_function
- 如果你的工作目录不是父目录的子目录,则可以像这样将父目录的完整路径添加到路径中
import sys
sys.path.append('/path/to/parent_directory')
# from parent_module import parent_function
确保将 parent_module
和 parent_function
替换为父模块和函数的名称。
通过将父目录添加到系统的 sys.path
列表中,你可以在 Python 代码中从父目录 import
模块。

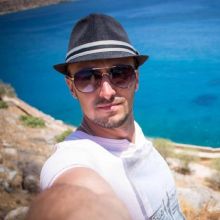