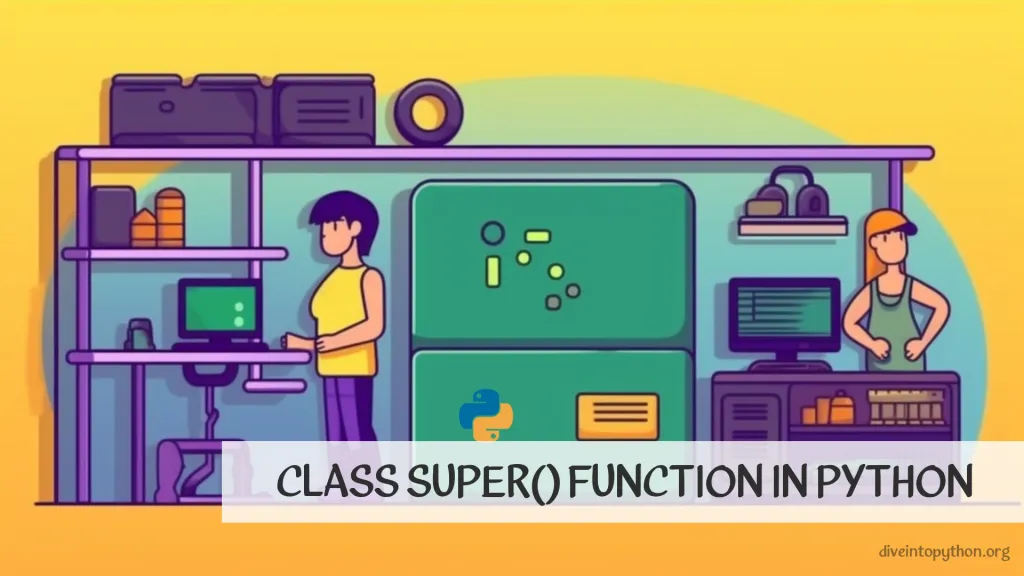
在本文中,我们将深入探讨 Python 超类的复杂性。通过掌握 Python 超类的基本要素,准备好释放 Python 开发的全部潜力。
了解 Python 的超类
super()
python 中的函数用于从子类中调用父类中的方法。super()
函数有助于在 Python 中的类之间创建易于维护和理解的层次结构。在 Python 3 中,super()
通常用于初始化抽象类。
在 Python 类中使用 super()
class MyParentClass:
def do_something(self):
print("Parent class doing something.")
class MyChildClass(MyParentClass):
def do_something(self):
super().do_something()
print("Child class doing something.")
child = MyChildClass()
child.do_something()
总之,super()
是 Python 中一个强大的函数,它提供了一种简洁明了的方式来从子类中调用父类方法。它有助于在类之间创建清晰的层次结构并维护简洁的代码。
Python 抽象类
抽象类是一个不能实例化且旨在被其他类子类化的类。super()
函数用于访问和调用 Python 中父类或超类中的方法。以下两个代码示例说明了 super()
在 Python 抽象类中的用法。
定义抽象类
import abc
class MyAbstractClass(metaclass=abc.ABCMeta):
def __init__(self):
super().__init__()
print("Initializing MyAbstractClass")
@abc.abstractmethod
def my_method(self):
pass
class MySubclass(MyAbstractClass):
def __init__(self):
super().__init__()
print("Initializing MySubclass")
def my_method(self):
print("Calling my_method in MySubclass")
my_obj = MySubclass()
my_obj.my_method()
# Output:
# Initializing MyAbstractClass
# Initializing MySubclass
# Calling my_method in MySubclass
在此示例中,我们定义了一个抽象类 MyAbstractClass
,其中包含一个抽象方法 my_method()
。该类有一个 __init__
方法,该方法使用 super()
调用超类的 __init__
方法。然后,我们定义一个子类 MySubclass
,该子类覆盖 __init__
方法并实现 my_method()
方法。当我们实例化 MySubclass
的一个对象时,将按顺序调用两个 __init__
方法,并调用 MySubclass
的 my_method()
方法。
定义父类
class MyParentClass:
def my_method(self):
print("Calling my_method in MyParentClass")
class MyChildClass(MyParentClass):
def my_method(self):
super().my_method()
print("Calling my_method in MyChildClass")
my_obj = MyChildClass()
my_obj.my_method()
# Output:
# Calling my_method in MyParentClass
# Calling my_method in MyChildClass
在此示例中,我们定义了一个父类 MyParentClass
,其中包含一个方法 my_method()
。然后,我们定义一个子类 MyChildClass
,该子类覆盖 my_method()
并使用 super()
调用父类中的相同方法。当我们实例化 MyChildClass
的一个对象时,首先调用父类中的 my_method()
方法,然后调用子类中的相同方法。
super() 在类继承中的作用
在 Python 中,super()
是一个内置函数,它通过继承提供对父类方法的访问。它用于在不显式命名父类的情况下调用父类的方法。它还用于 Python 3 抽象类中调用 super().__init__()
方法。
class Parent:
def __init__(self, name):
self.name = name
class Child(Parent):
def __init__(self, name, age):
super().__init__(name) # Call Parent class __init__ method
self.age = age
child = Child("John", 10)
print(child.name) # Output: John
在上面的示例中,Child
类继承 Parent
类,并使用 super()
函数初始化 name
属性。
from abc import ABC, abstractmethod
class Parent(ABC):
@abstractmethod
def calculate(self, x, y):
pass
class Child(Parent):
def __init__(self, name):
self.name = name
super().__init__() # Call Parent class __init__ method
def calculate(self, x, y):
return x + y
child = Child("John")
print(child.calculate(4, 5)) # Output: 9
在上面的示例中,Parent
类是一个抽象类。Child
类继承 Parent
类,并使用 super().__init__()
方法初始化父类。calculate()
方法在 Child
类中实现。
超类的强大功能
Python 中的 super()
函数用于从子类中调用超类的方法。它非常强大且有用,在适当使用时可以简化继承层次结构。
在 Python 3 中,super()
接受两个参数:第一个是调用 super()
的子类,第二个是子类实例或类本身。例如
class A:
def __init__(self):
print("I am in A's __init__")
class B(A):
def __init__(self):
super().__init__()
print("I am in B's __init__")
b = B()
# Output
# I am in A's __init__
# I am in B's __init__
在此示例中,super().__init__()
调用类 A
的 __init__()
方法,A
是 B
的超类。这样,当创建 B
的新对象时,A
和 B
都将被初始化。
另一个用例是包含 super().__init__()
的抽象类,在 __init__()
方法中
from abc import ABC, abstractmethod
class MyAbstractClass(ABC):
def __init__(self):
super().__init__()
@abstractmethod
def my_abstract_method(self):
pass
class MyClass(MyAbstractClass):
def __init__(self):
super().__init__()
def my_abstract_method(self):
print("I am implementing my abstract method")
c = MyClass()
在此示例中,MyAbstractClass
是一个抽象类,它定义了一个名为 my_abstract_method()
的 abstractmethod()
。MyClass
类继承自 MyAbstractClass
,并定义了 my_abstract_method()
的实现。当创建一个新的 MyClass
对象时,它的 __init__()
方法会调用 super().__init__()
,它会初始化抽象类 MyAbstractClass
。
总体而言,super()
函数是 Python 中一个强大的工具,它可以简化继承层次结构并简化对象初始化。
使用超类实现多重继承
Python 支持多重继承,这意味着一个类可以从多个父类继承。在这种情况下,super()
函数在管理调用父类方法的顺序方面发挥着至关重要的作用。在处理多重继承时,可以使用 super()
有效地导航方法解析顺序 (MRO)。
示例
class A:
def some_method(self):
print("Method from class A")
class B:
def some_method(self):
print("Method from class B")
class C(A, B):
def some_method(self):
super().some_method()
print("Method from class C")
obj = C()
obj.some_method()
输出
Method from class A
Method from class C
在此示例中,类 C
同时继承自 A
和 B
。super()
函数有助于在 MRO 中调用类 A
的方法,然后调用它自己的方法。
方法解析顺序 (MRO)
方法解析顺序 (MRO) 是多重继承中的一个关键概念。Python 使用 C3 线性化算法来确定调用方法的顺序。了解 MRO 可以帮助你预测 super()
在复杂继承层次结构中的作用。
示例
class A:
def some_method(self):
print("Method from class A")
class B(A):
def some_method(self):
print("Method from class B")
class C(A):
def some_method(self):
print("Method from class C")
class D(B, C):
def some_method(self):
super().some_method()
print("Method from class D")
obj = D()
obj.some_method()
输出
Method from class B
Method from class C
Method from class D
在此示例中,D
同时继承自 B
和 C
,MRO 确保了方法调用的正确顺序。
在你的文章中包含这些部分将全面了解 super()
在不同继承场景中的工作原理以及方法解析顺序在 Python 中的重要性。
使用超类的多级继承
多级继承是面向对象编程中的一种常见模式,其中一个子类继承自另一个子类。在这种情况下,super()
允许你访问和扩展父类的行为,而无需将你的代码紧密耦合到特定的类名。
示例
class Grandparent:
def speak(self):
print("Grandparent speaks")
class Parent(Grandparent):
def speak(self):
super().speak()
print("Parent speaks")
class Child(Parent):
def speak(self):
super().speak()
print("Child speaks")
obj = Child()
obj.speak()
输出
Grandparent speaks
Parent speaks
Child speaks
在此,super()
函数确保类层次结构的每一层都对最终行为做出贡献。

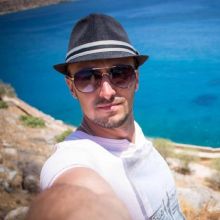